用链栈实现各项基本操作
时间: 2023-06-16 12:05:03 浏览: 92
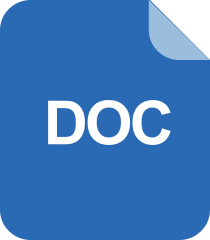
链栈及其操作
链栈是一种基于链表实现的栈结构。下面是链栈的各项基本操作的实现:
1. 初始化链栈
```
typedef struct Node{
int data;
struct Node* next;
}Node;
typedef struct Stack{
Node* top;
int size;
}Stack;
void InitStack(Stack* s){
s->top = NULL;
s->size = 0;
}
```
2. 判断栈是否为空
```
int IsEmpty(Stack* s){
return s->top == NULL;
}
```
3. 入栈
```
void Push(Stack* s, int x){
Node* node = (Node*)malloc(sizeof(Node));
node->data = x;
node->next = s->top;
s->top = node;
s->size++;
}
```
4. 出栈
```
int Pop(Stack* s){
if(IsEmpty(s)){
printf("栈为空,无法出栈!\n");
return -1;
}
Node* node = s->top;
int x = node->data;
s->top = node->next;
free(node);
s->size--;
return x;
}
```
5. 获取栈顶元素
```
int GetTop(Stack* s){
if(IsEmpty(s)){
printf("栈为空,无法获取栈顶元素!\n");
return -1;
}
return s->top->data;
}
```
6. 获取栈的大小
```
int GetSize(Stack* s){
return s->size;
}
```
上述代码实现了链栈的各项基本操作,可以通过调用这些函数来使用链栈。
阅读全文
相关推荐
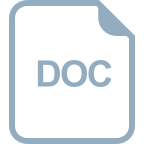
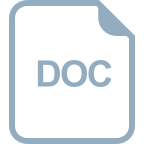
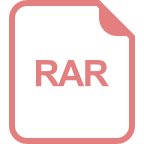
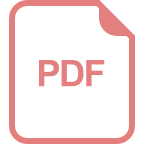
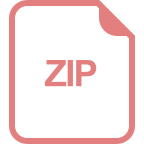
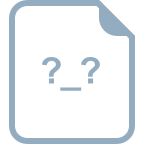








