c++实现链栈并实现链栈的基本查询功能
时间: 2023-08-28 07:36:05 浏览: 92
好的,下面是 C++ 实现链栈并实现基本查询功能的示例代码:
```c++
#include <iostream>
using namespace std;
struct Node {
int data;
Node* next;
};
class Stack {
private:
Node* top; // 链栈的栈顶指针
public:
Stack() { top = NULL; } // 构造函数,将栈顶指针初始化为 NULL
~Stack(); // 析构函数,释放链栈的所有节点内存空间
bool isEmpty() const { return top == NULL; } // 判断链栈是否为空
void push(int item); // 入栈
int pop(); // 出栈
int peek() const; // 获取栈顶元素
};
Stack::~Stack() {
Node* p;
while (top != NULL) {
p = top;
top = top->next;
delete p;
}
}
void Stack::push(int item) {
Node* newNode = new Node;
newNode->data = item;
newNode->next = top;
top = newNode;
}
int Stack::pop() {
if (isEmpty()) {
cout << "栈空,不能出栈!" << endl;
return 0;
}
Node* p = top;
int item = p->data;
top = top->next;
delete p;
return item;
}
int Stack::peek() const {
if (isEmpty()) {
cout << "栈空,没有栈顶元素!" << endl;
return 0;
}
return top->data;
}
int main() {
Stack s;
s.push(1);
s.push(2);
s.push(3);
cout << "栈顶元素:" << s.peek() << endl;
int item = s.pop();
cout << "出栈元素:" << item << endl;
cout << "栈顶元素:" << s.peek() << endl;
item = s.pop();
cout << "出栈元素:" << item << endl;
cout << "栈顶元素:" << s.peek() << endl;
item = s.pop();
cout << "出栈元素:" << item << endl;
cout << "栈是否为空:" << (s.isEmpty() ? "是" : "否") << endl;
return 0;
}
```
代码中定义了一个 `Node` 结构体表示链栈中的节点,使用一个 `top` 指针来表示链栈的栈顶。根据链栈的特点,入栈操作只需要在链表头部插入一个新的节点,出栈操作只需要删除链表头部的节点即可。`peek` 操作用于获取栈顶元素,判断链栈是否为空只需要判断 `top` 是否为 `NULL` 即可。
阅读全文
相关推荐





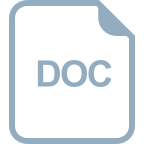









