C#中的多态是什么?如何实现多态?
时间: 2023-08-28 11:20:26 浏览: 301
在C#中,多态(Polymorphism)是一种面向对象编程的特性,允许通过基类的引用来调用派生类中的方法,实现了一个抽象类型的多种不同形态。
多态可以通过以下几种方式来实现:
1. 虚方法(Virtual Methods):在基类中声明一个虚方法,并在派生类中对其进行重写。基类的引用可以指向派生类的实例,并调用派生类重写的方法。
```csharp
public class Animal
{
public virtual void MakeSound()
{
Console.WriteLine("The animal makes a sound");
}
}
public class Dog : Animal
{
public override void MakeSound()
{
Console.WriteLine("The dog barks");
}
}
public class Cat : Animal
{
public override void MakeSound()
{
Console.WriteLine("The cat meows");
}
}
Animal animal = new Dog();
animal.MakeSound(); // Output: "The dog barks"
animal = new Cat();
animal.MakeSound(); // Output: "The cat meows"
```
2. 抽象类和抽象方法(Abstract Classes and Methods):抽象类是不能被实例化的,只能被继承。抽象方法在抽象类中声明,要求派生类对其进行实现。
```csharp
public abstract class Shape
{
public abstract double CalculateArea();
}
public class Rectangle : Shape
{
public double Width { get; set; }
public double Height { get; set; }
public override double CalculateArea()
{
return Width * Height;
}
}
public class Circle : Shape
{
public double Radius { get; set; }
public override double CalculateArea()
{
return Math.PI * Radius * Radius;
}
}
Shape shape = new Rectangle { Width = 5, Height = 3 };
Console.WriteLine(shape.CalculateArea()); // Output: 15
shape = new Circle { Radius = 2 };
Console.WriteLine(shape.CalculateArea()); // Output: approximately 12.57
```
3. 接口(Interfaces):接口定义了一组方法和属性的契约,类可以实现一个或多个接口。通过接口,可以实现多态行为。
```csharp
public interface IPlayable
{
void Play();
}
public class MusicPlayer : IPlayable
{
public void Play()
{
Console.WriteLine("Playing music");
}
}
public class VideoPlayer : IPlayable
{
public void Play()
{
Console.WriteLine("Playing video");
}
}
IPlayable player = new MusicPlayer();
player.Play(); // Output: "Playing music"
player = new VideoPlayer();
player.Play(); // Output: "Playing video"
```
以上是在C#中实现多态的几种常见方式。多态让代码更具灵活性和可扩展性,使得我们可以以一种通用的方式处理不同类型的对象。
阅读全文
相关推荐
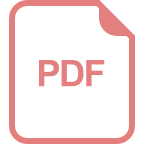
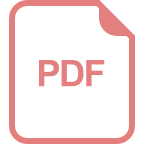
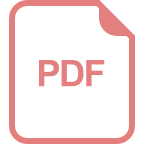















