客户端A发送报文给服务端B,服务端B转发报文给服务端C,服务端C接收到后回复给B,B回复给A,B使用netty实现的完整代码
时间: 2024-12-14 07:16:29 浏览: 1
客户端A发送报文到服务端B并由B转发的过程通常涉及到网络通信库的使用,比如Netty。Netty是一个高性能、异步事件驱动的网络应用框架。以下是一个简单的Netty示例,展示了如何在服务端B中接收请求、转发到服务端C,然后将C的响应返回给客户端A:
```java
// 导入必要的依赖
import io.netty.bootstrap.ServerBootstrap;
import io.netty.channel.ChannelFuture;
import io.netty.channel.ChannelInitializer;
import io.netty.channel.EventLoopGroup;
import io.netty.channel.nio.NioEventLoopGroup;
import io.netty.channel.socket.SocketChannel;
import io.netty.channel.socket.nio.NioServerSocketChannel;
public class ServiceServer {
private static final int PORT = 8080; // 服务器B监听的端口
public static void main(String[] args) throws Exception {
// 创建工作线程组
EventLoopGroup bossGroup = new NioEventLoopGroup();
EventLoopGroup workerGroup = new NioEventLoopGroup();
try {
// 绑定服务器
ServerBootstrap b = new ServerBootstrap();
b.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.childHandler(new ChannelInitializer<SocketChannel>() {
@Override
protected void initChannel(SocketChannel ch) throws Exception {
ch.pipeline().addLast(new RequestForwarder("localhost", 8081)); // 转发到服务端C
ch.pipeline().addLast(new ResponseHandler()); // 收到C的回应后处理
}
});
// 启动服务器
ChannelFuture f = b.bind(PORT).sync();
// 等待关闭
f.channel().closeFuture().sync();
} finally {
// 关闭线程池
workerGroup.shutdownGracefully();
bossGroup.shutdownGracefully();
}
}
// 相关处理器类,如RequestForwarder用于封装和转发请求,ResponseHandler用于处理和回复响应
private static class RequestForwarder extends ChannelInboundHandlerAdapter {
private String targetHost;
private int targetPort;
public RequestForwarder(String targetHost, int targetPort) {
this.targetHost = targetHost;
this.targetPort = targetPort;
}
@Override
public void channelRead(ChannelHandlerContext ctx, Object msg) {
// 对接收到的消息进行处理,然后通过ctx writeAndFlush到下一个通道(服务端C)
ctx.writeAndFlush(msg, ctx.newPromise()).addListener((Future<Void>) future -> {
if (!future.isSuccess()) {
ctx.close(); // 如果转发失败,关闭连接
}
});
}
@Override
public void exceptionCaught(ChannelHandlerContext ctx, Throwable cause) {
cause.printStackTrace();
ctx.close(); // 发生异常时关闭连接
}
}
// 类似于RequestForwarder,处理从C收到的响应
private static class ResponseHandler extends ChannelInboundHandlerAdapter {
@Override
public void channelRead(ChannelHandlerContext ctx, Object msg) {
// 这里假设msg就是从C返回的数据,将其包装成响应并回送给客户端A
// 可能会包含序列化和反序列化步骤,实际操作取决于数据结构
// 假设data是msg内容,ctx.writeAndFlush(data);
ctx.close(); // 非常简化,这里仅作示例,实际应用可能会有更复杂的业务逻辑处理
}
}
}
```
阅读全文
相关推荐
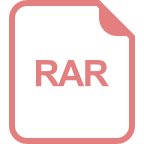
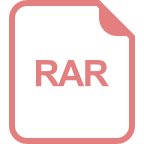
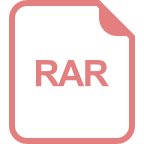






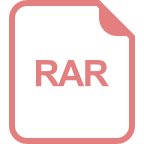








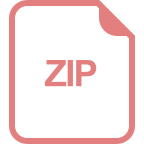
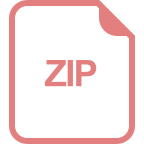