socket客户端A发送报文给Socket服务端B,服务端B转发给Socket服务端C,服务端C回复报文给B,B回复给A,A、B、C使用netty实现的代码
时间: 2024-12-14 19:15:08 浏览: 4
在Netty框架下,你可以使用ChannelInboundHandler接口和事件驱动的方式来构建这样的客户端和服务端通信模型。首先,我们来看一下基本的步骤:
**服务端B接收并转发消息**
```java
// 定义一个ChannelInboundHandler
public class ForwardingHandler extends ChannelInboundHandlerAdapter {
@Override
public void channelRead(ChannelHandlerContext ctx, Object msg) throws Exception {
// 获取目标服务器C的连接信息
String serverCAddress = ...; // 根据业务规则获取地址
// 创建一个新的ChannelFuture用于异步发送到C
ChannelPromise promise = ctx.newPromise();
Bootstrap b = new Bootstrap(); // 创建一个新的Bootstrap实例用于建立连接
b.group(ctx.channel().eventLoop())
.channel(NioSocketChannel.class)
.handler(new ChannelInitializer<SocketChannel>() {
@Override
protected void initChannel(SocketChannel ch) throws Exception {
ch.pipeline().addLast(promise);
// 添加转发到C的处理器
ch.pipeline().addLast(new ToServerCHandler(serverCAddress));
}
});
// 异步连接到C并发送消息
b.connect(serverCAddress).syncUninterruptibly();
// 如果连接成功,通知原请求者A消息已转发
if (promise.isSuccess()) {
ctx.writeAndFlush(msg); // 将接收到的消息回传给A
} else {
// 处理错误
handleException(ctx, promise.cause());
}
}
private void handleException(ChannelHandlerContext ctx, Throwable cause) {
// 错误处理逻辑
...
}
}
```
**服务端C接收并回复消息**
```java
// 客户端C的处理类ToServerCHandler
public class ToServerCHandler extends ChannelInboundHandlerAdapter {
private final String targetAddress;
public ToServerCHandler(String targetAddress) {
this.targetAddress = targetAddress;
}
@Override
public void channelActive(ChannelHandlerContext ctx) {
super.channelActive(ctx);
// 发送确认消息给B
ctx.writeAndFlush("Message received from A, acknowledged to B");
}
@Override
public void channelRead(ChannelHandlerContext ctx, Object msg) {
// 处理来自A的消息
...
// 回复消息给A
ctx.writeAndFlush(replyMsg);
}
}
```
**客户端A的交互**
```java
// 客户端A连接到服务器B
Bootstrap clientA = new Bootstrap();
clientA.group(...).channel(NioSocketChannel.class).handler(new ClientAHandler());
ChannelFuture connectFuture = clientA.connect(BServerAddress);
connectFuture.sync();
// 发送消息
connectFuture.channel().writeAndFlush(requestMsg);
// 监听回复
connectFuture.channel().pipeline().get(ForwardingHandler.class).channelReadListener(this::handleResponse);
```
在这个例子中,`handleResponse`方法可以用于处理从服务器B返回的消息。
阅读全文
相关推荐
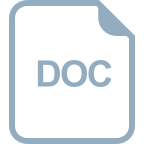
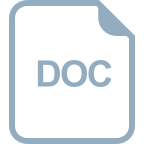
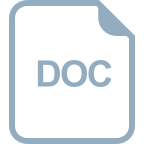

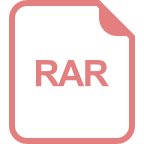



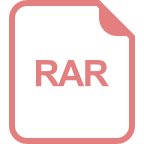
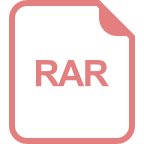
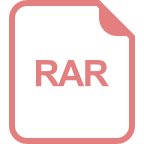
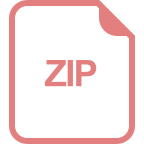






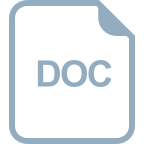