socket客户端A发送报文给Socket服务端B,服务端B将报文转发给Socket服务端C,服务端C回复报文给B,B再将报文回复给A,A和C之间没有直连,只能通过B做中转,服务端B使用netty实现的代码以及转发报文的主要代码
时间: 2024-12-14 14:20:50 浏览: 1
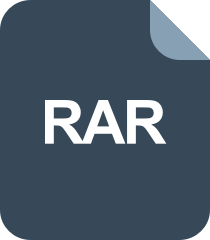
Socket测试工具 设置服务端,发送socket报文
在Netty框架中,为了实现这种代理服务器模式,你可以创建一个ServerHandler,它接收来自客户端A的请求,然后将消息封装到ChannelHandlerContext中,并通过channel.writeAndFlush()方法转发到另一个目标通道(连接着服务端C)。这里是一个简化版的示例代码:
```java
import io.netty.bootstrap.ServerBootstrap;
import io.netty.channel.ChannelFuture;
import io.netty.channel.ChannelInitializer;
import io.netty.channel.ChannelOption;
import io.netty.channel.EventLoopGroup;
import io.netty.channel.nio.NioEventLoopGroup;
import io.netty.channel.socket.SocketChannel;
import io.netty.channel.socket.nio.NioServerSocketChannel;
public class ForwardingServer {
public static void main(String[] args) throws Exception {
// 创建两个工作线程组,一个用于服务器接收,一个用于转发
EventLoopGroup bossGroup = new NioEventLoopGroup();
EventLoopGroup workerGroup = new NioEventLoopGroup();
try {
ServerBootstrap b = new ServerBootstrap();
b.group(bossGroup, workerGroup)
.channel(NioServerSocketChannel.class)
.option(ChannelOption.SO_BACKLOG, 100)
.childHandler(new ChannelInitializer<SocketChannel>() {
@Override
protected void initChannel(SocketChannel ch) throws Exception {
ch.pipeline().addLast(new ForwardingHandler());
}
});
// 绑定并启动服务器
ChannelFuture f = b.bind(8080).sync();
// 等待关闭
f.channel().closeFuture().sync();
} finally {
bossGroup.shutdownGracefully();
workerGroup.shutdownGracefully();
}
}
private static class ForwardingHandler extends ChannelInboundHandlerAdapter {
@Override
public void channelRead(ChannelHandlerContext ctx, Object msg) throws Exception {
// 接收到客户端的消息后,可以将其包装成适合转发的形式,如ByteBuf或者Object
// 示例中假设msg是一个String
String message = (String) msg;
// 根据实际需要找到目标服务端C的连接信息,比如从配置文件获取
// 这里仅作演示,假设有一个目标地址targetAddress
String targetAddress = ...;
// 转发消息到目标服务器C
ctx.channel().writeAndFlush(message, ctx.newPromise()); // 使用异步操作保证非阻塞
// 如果你想添加一些日志或者错误处理,可以在这里的回调函数中实现
}
// 其他ChannelInboundHandlerAdapter的方法...
}
}
```
在这个例子中,`ForwardingHandler`负责接收来自A的报文,然后将报文发送到`targetAddress`指定的目标服务器C。`channelWriteAndFlush()`会异步地将消息发送出去,不会阻塞当前的事件循环。
阅读全文
相关推荐
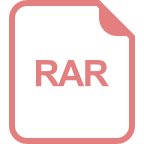
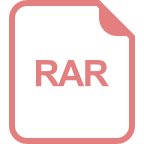















