一个Android GATT客户端访问数据,一个Android GATT服务端提供数据
时间: 2023-11-11 22:09:19 浏览: 203
在Android中,可以通过使用BluetoothGatt来实现GATT客户端和服务端的通信。
对于GATT客户端,你需要执行以下步骤:
1. 获取BluetoothAdapter实例并检查设备是否支持BLE。
2. 扫描并连接到GATT服务端。
3. 获取BluetoothGatt实例并连接到GATT服务端。
4. 搜索GATT服务和特征。
5. 读取或写入GATT特征的值。
对于GATT服务端,你需要执行以下步骤:
1. 获取BluetoothManager和BluetoothAdapter实例并检查设备是否支持BLE。
2. 创建BluetoothGattServer实例并添加服务和特征。
3. 等待客户端连接。
4. 处理客户端请求,并响应读取或写入特征值的请求。
下面是一个简单的GATT客户端和服务端的示例代码:
GATT客户端:
```java
BluetoothManager bluetoothManager = (BluetoothManager) getSystemService(Context.BLUETOOTH_SERVICE);
BluetoothAdapter bluetoothAdapter = bluetoothManager.getAdapter();
// 检查设备是否支持BLE
if (bluetoothAdapter == null || !bluetoothAdapter.isEnabled()) {
Log.e(TAG, "Bluetooth is not available.");
return;
}
// 扫描并连接到GATT服务端
bluetoothAdapter.startLeScan(mLeScanCallback);
mBluetoothGatt = device.connectGatt(this, false, mGattCallback);
// 获取BluetoothGatt实例并连接到GATT服务端
BluetoothDevice device = bluetoothAdapter.getRemoteDevice(address);
mBluetoothGatt = device.connectGatt(this, false, mGattCallback);
// 搜索GATT服务和特征
mBluetoothGatt.discoverServices();
// 读取GATT特征的值
BluetoothGattCharacteristic characteristic = mBluetoothGatt.getService(serviceUuid)
.getCharacteristic(characteristicUuid);
mBluetoothGatt.readCharacteristic(characteristic);
// 写入GATT特征的值
BluetoothGattCharacteristic characteristic = mBluetoothGatt.getService(serviceUuid)
.getCharacteristic(characteristicUuid);
characteristic.setValue(value);
mBluetoothGatt.writeCharacteristic(characteristic);
```
GATT服务端:
```java
BluetoothManager bluetoothManager = (BluetoothManager) getSystemService(Context.BLUETOOTH_SERVICE);
BluetoothAdapter bluetoothAdapter = bluetoothManager.getAdapter();
// 检查设备是否支持BLE
if (bluetoothAdapter == null || !bluetoothAdapter.isEnabled()) {
Log.e(TAG, "Bluetooth is not available.");
return;
}
// 创建BluetoothGattServer实例并添加服务和特征
mGattServer = bluetoothManager.openGattServer(this, mGattServerCallback);
BluetoothGattService service = new BluetoothGattService(serviceUuid, BluetoothGattService.SERVICE_TYPE_PRIMARY);
BluetoothGattCharacteristic characteristic = new BluetoothGattCharacteristic(characteristicUuid,
BluetoothGattCharacteristic.PROPERTY_READ | BluetoothGattCharacteristic.PROPERTY_WRITE,
BluetoothGattCharacteristic.PERMISSION_READ | BluetoothGattCharacteristic.PERMISSION_WRITE);
service.addCharacteristic(characteristic);
mGattServer.addService(service);
// 等待客户端连接
mGattServerCallback.onConnectionStateChange(gatt, status, newState);
// 处理客户端请求,并响应读取或写入特征值的请求
mGattServerCallback.onCharacteristicReadRequest(gatt, requestId, offset, characteristic);
mGattServerCallback.onCharacteristicWriteRequest(gatt, requestId, characteristic, preparedWrite, responseNeeded, offset, value);
```
这只是一个简单的示例,实际应用中需要根据具体的需求进行修改和完善。
阅读全文
相关推荐
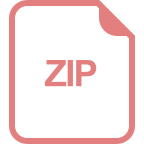
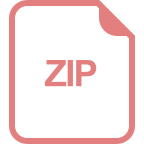
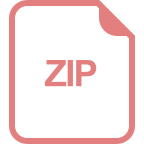
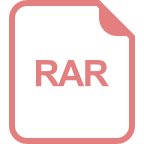
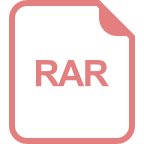
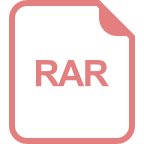
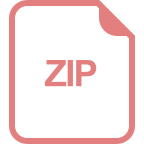
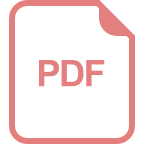
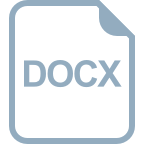
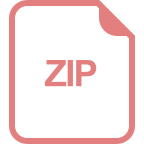
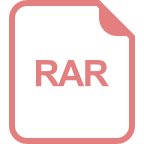
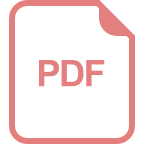
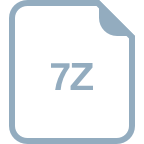
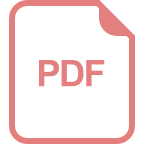
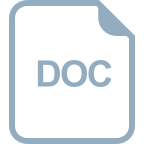
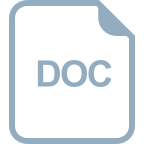