Python有哪些库可以帮助创建彩色爱心图案?
时间: 2024-09-29 17:12:59 浏览: 40
在Python中,有一些库可以帮助创建彩色爱心图案,其中最流行的是`ansiwrap`和`colorama`,这两个库配合使用可以让文本输出具备颜色和样式。`ansiwrap`用于处理终端宽度,而`colorama`则负责跨平台地支持ANSI转义码,使得输出支持Windows系统的终端颜色。
下面是一个例子,展示了如何结合这两个库来创建带颜色的爱心:
```python
from ansiwrap import wrap
from colorama import Fore, Back, Style
def colored_heart(text, fore_color, back_color):
wrapped_text = wrap(text, width=30)
colored_line = f"{Fore.{fore_color}}{Back.{back_color}}{wrapped_text}{Style.RESET_ALL}"
return colored_line
# 示例,创建红色爱心在黑色背景上
fore_color = "RED"
back_color = "BLACK"
text = "\u2764\ufe0f" # 爱心符号
colored_heart(text, fore_color, back_color)
相关问题
如何使用Python的图形库来创建彩色爱心图像?
Python 的图形库很多,如 `PIL`(Python Imaging Library),`matplotlib` 和 `pygame` 等。这里以 `Pillow`(PIL的一个分支,支持更多的现代特性)为例,介绍如何使用它创建彩色爱心图像。
首先,你需要安装 `Pillow` 库,如果还没有安装,可以使用 `pip install pillow` 进行安装。
```python
from PIL import Image, ImageDraw
# 创建一个新的白色背景图像
image = Image.new("RGB", (200, 200), "white")
# 创建一个绘图工具
draw = ImageDraw.Draw(image)
# 定义心形的路径
def heart_path(x, y):
path = [(x, y), (x - 10, y - 5), (x - 20, y - 15), (x - 10, y - 20),
(x, y - 18), (x + 5, y - 10), (x + 15, y - 5), (x + 20, y)]
return path
# 绘制彩色爱心
fill_colors = [(255, 0, 0)] # 红色填充
outline_color = (0, 0, 255) # 蓝色描边
for x in range(90, image.width - 90, 10):
for y in range(90, image.height - 90, 10):
path = heart_path(x, y)
fill = fill_colors if y % 2 == 0 else None # 可调整颜色模式
outline = outline_color
draw.polygon(path, fill=fill, outline=outline)
# 显示和保存图片
image.show()
image.save("colored_heart.png")
```
这段代码会创建一个红色填充蓝色描边的爱心图案,并将其保存为 PNG 文件。你可以通过修改 `fill_colors` 和 `outline_color` 来改变颜色。
Python有没有现成的库可以生成彩色爱心图像?
Python有一个名为"PIL"(Pillow)的流行图像处理库,它可以用来生成各种图像,包括彩色的心形图案。你可以使用PIL的绘图模块如`ImageDraw`和`ImageFont`来绘制形状,并设置颜色。另外,还有一些专门用于图形艺术创作的库,比如`pygame`,它也有能力生成复杂的图形,包括心形。
如果你想要更简单易用的API,还有第三方库`pycairo`或`svgwrite`,它们可以利用更高级的功能生成矢量图形,其中包括心形。这些库通常需要一些基础的图形设计知识,但能够创建高质量、可缩放的图像。
要开始制作彩色爱心图像,首先需要安装相应库,然后编写一个简单的脚本来绘制。这里是一个简短的例子:
```python
from PIL import Image, ImageDraw
def draw_heart(image_size=(200, 200), fill_color='red'):
img = Image.new('RGB', image_size, 'white')
draw = ImageDraw.Draw(img)
heart_shape = [(78, 45), (95, 62), (108, 73), (115, 78),
(116, 76), (114, 66), (108, 63), (99, 65),
(90, 70), (80, 67), (71, 71), (62, 68),
(54, 65), (47, 62), (41, 58), (36, 55),
(32, 53), (28, 51), (24, 49)]
draw.polygon(heart_shape, fill=fill_color)
return img
# 生成红色爱心图像并保存
heart_img = draw_heart()
heart_img.save("heart.png")
```
阅读全文
相关推荐
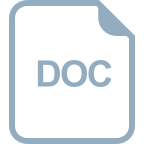
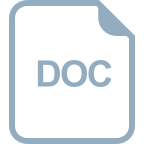
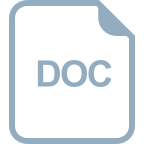













