python随机抽样代码
时间: 2024-10-21 11:15:00 浏览: 15
在Python中,你可以使用内置的random模块来进行各种类型的随机抽样。这里有一些常见的例子:
1. **从列表中随机选择一个元素**:
```python
import random
my_list = [1, 2, 3, 4, 5]
random_element = random.choice(my_list)
print(random_element)
```
2. **无放回随机抽样(样本大小小于列表长度)**:
```python
import random
def sample_without_replacement(lst, k):
return random.sample(lst, k)
sample = sample_without_replacement(my_list, 3)
print(sample)
```
3. **有放回随机抽样(每次抽取后都放回原列表)**:
```python
def sample_with_replacement(lst, k):
return [random.choice(lst) for _ in range(k)]
sample = sample_with_replacement(my_list, 5)
print(sample)
```
4. **随机打乱列表顺序**:
```python
random.shuffle(my_list)
print(my_list)
```
相关问题
python随机抽样
在Python中,你可以使用`random`模块来进行随机抽样。下面是几种常见的随机抽样方法的示例代码:
1. 随机抽取列表中的一个元素:
```python
import random
my_list = [1, 2, 3, 4, 5]
random_element = random.choice(my_list)
print(random_element)
```
2. 随机抽取列表中的多个元素(样本):
```python
import random
my_list = [1, 2, 3, 4, 5]
sample = random.sample(my_list, 3) # 随机抽取3个元素作为样本
print(sample)
```
3. 随机打乱列表顺序:
```python
import random
my_list = [1, 2, 3, 4, 5]
random.shuffle(my_list) # 随机打乱列表顺序
print(my_list)
```
这些示例代码展示了如何使用`random`模块来进行随机抽样。你可以根据具体需求选择适合的方法。
python 随机抽样
你可以使用Python的random模块来进行随机抽样。具体来说,你可以使用random.sample()函数来从给定的序列中随机抽取指定数量的元素。以下是一个例子:
```python
import random
# 创建一个列表作为示例
my_list = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
# 从列表中随机抽取3个元素
sample = random.sample(my_list, 3)
print(sample)
```
这段代码将会从`my_list`中随机抽取3个不重复的元素,并将结果存储在`sample`变量中。你可以根据需要调整抽样的数量和输入的序列。
阅读全文
相关推荐
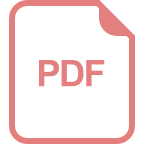
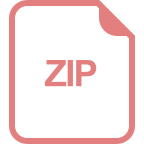
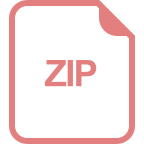













