请使用C++语言编写:应用宽度优先搜索求解重排九宫问题
时间: 2023-06-24 16:08:48 浏览: 83
以下是使用C++语言编写的应用宽度优先搜索求解重排九宫问题的代码:
```c++
#include <iostream>
#include <queue>
#include <unordered_set>
using namespace std;
//定义九宫格状态
struct State {
int nums[9]; //九宫格状态
int zero_pos; //空格所在的位置
string path; //记录路径
};
//判断九宫格状态是否已经达到目标状态
bool is_goal(State& s) {
for(int i=0; i<8; i++) {
if(s.nums[i] != i+1) {
return false;
}
}
return (s.nums[8] == 0);
}
//交换九宫格状态中的两个数
State swap_num(State& s, int pos1, int pos2) {
State new_state = s;
int temp = new_state.nums[pos1];
new_state.nums[pos1] = new_state.nums[pos2];
new_state.nums[pos2] = temp;
return new_state;
}
//宽度优先搜索求解重排九宫问题
string bfs(State& start_state) {
queue<State> q;
unordered_set<string> visited; //用哈希表记录已经访问过的状态
q.push(start_state);
visited.insert(string(start_state.nums, start_state.nums+9)); //将初始状态加入已访问状态
while(!q.empty()) {
State cur_state = q.front();
q.pop();
//尝试移动空格
if(cur_state.zero_pos >= 3) { //空格可以向上移动
State new_state = swap_num(cur_state, cur_state.zero_pos, cur_state.zero_pos-3);
if(visited.find(string(new_state.nums, new_state.nums+9)) == visited.end()) { //新状态未访问过
new_state.zero_pos = cur_state.zero_pos-3;
new_state.path = cur_state.path + "u";
if(is_goal(new_state)) { //新状态为目标状态
return new_state.path;
}
q.push(new_state); //将新状态加入队列
visited.insert(string(new_state.nums, new_state.nums+9)); //将新状态加入已访问状态
}
}
if(cur_state.zero_pos <= 5) { //空格可以向下移动
State new_state = swap_num(cur_state, cur_state.zero_pos, cur_state.zero_pos+3);
if(visited.find(string(new_state.nums, new_state.nums+9)) == visited.end()) { //新状态未访问过
new_state.zero_pos = cur_state.zero_pos+3;
new_state.path = cur_state.path + "d";
if(is_goal(new_state)) { //新状态为目标状态
return new_state.path;
}
q.push(new_state); //将新状态加入队列
visited.insert(string(new_state.nums, new_state.nums+9)); //将新状态加入已访问状态
}
}
if(cur_state.zero_pos % 3 != 0) { //空格可以向左移动
State new_state = swap_num(cur_state, cur_state.zero_pos, cur_state.zero_pos-1);
if(visited.find(string(new_state.nums, new_state.nums+9)) == visited.end()) { //新状态未访问过
new_state.zero_pos = cur_state.zero_pos-1;
new_state.path = cur_state.path + "l";
if(is_goal(new_state)) { //新状态为目标状态
return new_state.path;
}
q.push(new_state); //将新状态加入队列
visited.insert(string(new_state.nums, new_state.nums+9)); //将新状态加入已访问状态
}
}
if(cur_state.zero_pos % 3 != 2) { //空格可以向右移动
State new_state = swap_num(cur_state, cur_state.zero_pos, cur_state.zero_pos+1);
if(visited.find(string(new_state.nums, new_state.nums+9)) == visited.end()) { //新状态未访问过
new_state.zero_pos = cur_state.zero_pos+1;
new_state.path = cur_state.path + "r";
if(is_goal(new_state)) { //新状态为目标状态
return new_state.path;
}
q.push(new_state); //将新状态加入队列
visited.insert(string(new_state.nums, new_state.nums+9)); //将新状态加入已访问状态
}
}
}
return ""; //无解
}
int main() {
State start_state = {{2, 8, 3, 1, 0, 4, 7, 6, 5}, 4, ""}; //初始状态
string path = bfs(start_state);
if(path == "") { //无解
cout << "No solution." << endl;
} else {
cout << "Solution: " << path << endl;
}
return 0;
}
```
该代码定义了一个九宫格状态的结构体 `State`,其中包含九宫格状态、空格所在位置和记录路径。使用队列和哈希表记录已经访问过的状态,在宽度优先搜索过程中,尝试向上、下、左、右四个方向移动空格,生成新的状态,并将新的状态加入队列和哈希表中。当新状态为目标状态时,返回路径。如果搜索完整个状态空间都没有找到解,则返回无解。
阅读全文
相关推荐


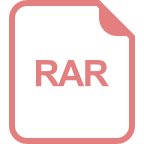
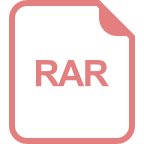
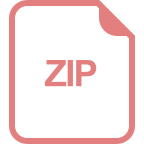
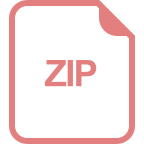
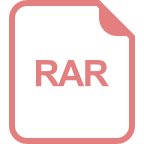
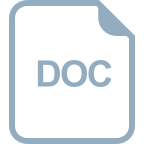
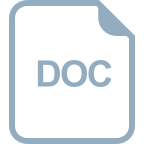

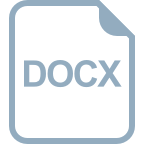