请使用C++语言应用宽度优先搜索求解重排九宫问题
时间: 2023-06-24 17:09:03 浏览: 61
好的,以下是使用C++语言应用宽度优先搜索求解重排九宫问题的代码:
```cpp
#include <iostream>
#include <queue>
#include <unordered_set>
#include <array>
using namespace std;
// 定义九宫格的大小
const int kSize = 9;
// 定义初始状态和目标状态
const array<int, kSize> kInitial = {1, 2, 3, 4, 5, 6, 0, 7, 8};
const array<int, kSize> kTarget = {1, 2, 3, 4, 5, 6, 7, 0, 8};
// 定义状态结构体
struct State {
array<int, kSize> board; // 当前状态的九宫格
int space_index; // 空格子的下标
bool operator==(const State& other) const {
return board == other.board;
}
};
// 定义哈希函数
namespace std {
template<>
struct hash<State> {
size_t operator()(const State& s) const {
size_t h = 0;
for (auto i : s.board) {
h += hash<int>()(i);
}
return h;
}
};
}
// 定义广度优先搜索函数
int bfs(State start) {
// 定义队列和已访问状态的哈希表
queue<State> q;
unordered_set<State> visited;
// 将初始状态加入队列和已访问状态的哈希表
q.push(start);
visited.insert(start);
// 定义步数
int steps = 0;
// 开始搜索
while (!q.empty()) {
// 遍历当前层的所有状态
int size = q.size();
for (int i = 0; i < size; i++) {
State current = q.front();
q.pop();
// 如果当前状态已经是目标状态,返回当前步数
if (current.board == kTarget) {
return steps;
}
// 找到空格子的位置
int space = current.space_index;
// 判断空格子能否向上移动
if (space >= 3) {
State next = current;
swap(next.board[space], next.board[space - 3]);
next.space_index = space - 3;
if (visited.count(next) == 0) {
q.push(next);
visited.insert(next);
}
}
// 判断空格子能否向下移动
if (space <= 5) {
State next = current;
swap(next.board[space], next.board[space + 3]);
next.space_index = space + 3;
if (visited.count(next) == 0) {
q.push(next);
visited.insert(next);
}
}
// 判断空格子能否向左移动
if (space % 3 != 0) {
State next = current;
swap(next.board[space], next.board[space - 1]);
next.space_index = space - 1;
if (visited.count(next) == 0) {
q.push(next);
visited.insert(next);
}
}
// 判断空格子能否向右移动
if (space % 3 != 2) {
State next = current;
swap(next.board[space], next.board[space + 1]);
next.space_index = space + 1;
if (visited.count(next) == 0) {
q.push(next);
visited.insert(next);
}
}
}
// 增加步数
steps++;
}
// 如果搜索完整个状态空间都没有找到目标状态,返回-1
return -1;
}
int main() {
// 构造初始状态
State start = {kInitial, 6};
// 求解重排九宫问题
int steps = bfs(start);
// 输出结果
if (steps == -1) {
cout << "无解" << endl;
} else {
cout << "最少需要 " << steps << " 步" << endl;
}
return 0;
}
```
该代码中,我们使用了STL中的queue和unordered_set来实现广度优先搜索,并使用哈希表来记录已访问的状态,以避免重复搜索。在搜索时,我们采用了宽度优先搜索的策略,先遍历当前层的所有状态,再遍历下一层的所有状态。具体实现中,我们在遍历当前状态时,依次尝试将空格子向上、向下、向左、向右移动,得到新的状态,并判断是否已经访问过,如果没有访问过,则加入队列和已访问状态的哈希表中。当遍历到目标状态时,返回当前步数即可。
需要注意的是,由于重排九宫问题可能存在无解的情况,因此在搜索完整个状态空间后仍未找到目标状态时,需要返回-1表示无解。
相关推荐
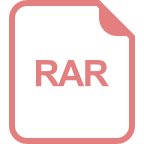
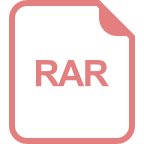














