编写一个程序,使用循环链表完成约瑟夫问题求解,使用C++
时间: 2023-12-03 12:44:14 浏览: 83
下面是使用循环链表完成约瑟夫问题求解的C++程序:
```c++
#include <iostream>
using namespace std;
// 定义循环链表结点
struct Node {
int val;
Node* next;
Node(int v) : val(v), next(NULL) {}
};
// 求解约瑟夫问题
int josephus(int n, int m) {
// 构建循环链表
Node* head = new Node(1);
Node* cur = head;
for (int i = 2; i <= n; i++) {
cur->next = new Node(i);
cur = cur->next;
}
cur->next = head;
// 模拟出圈过程
cur = head;
while (cur->next != cur) {
// 找到要出圈的结点的前一个结点
for (int i = 1; i < m; i++) {
cur = cur->next;
}
// 删除要出圈的结点
Node* tmp = cur->next;
cur->next = tmp->next;
delete tmp;
}
// 返回最后一个结点的编号
int res = cur->val;
delete cur;
return res;
}
int main() {
int n = 5, m = 2;
int res = josephus(n, m);
cout << "The last person's number is " << res << endl;
return 0;
}
```
在上面的程序中,我们先构建了一个包含n个结点的循环链表,然后进行出圈操作,直到最后只剩下一个结点,返回该结点的编号即可。
阅读全文
相关推荐
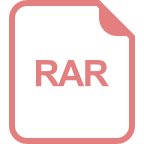
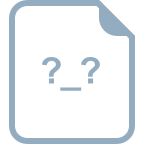


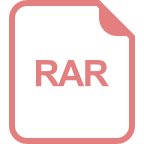
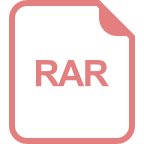
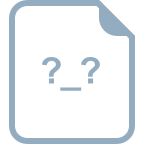
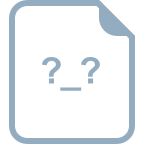
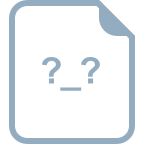
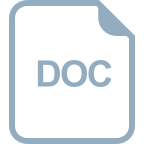
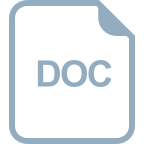






