继承构造函数(inheritance constructors)
时间: 2023-10-23 18:12:43 浏览: 49
继承构造函数是 C++11 中的一个新特性,它可以让派生类继承基类的构造函数,从而避免在派生类中重复定义基类构造函数的代码。通过继承构造函数,派生类可以直接使用基类的构造函数,而不必重新实现一遍。
继承构造函数的语法非常简单,只需要使用 using 关键字声明基类构造函数即可,如下所示:
```c++
class Derived : public Base {
public:
using Base::Base; // 继承基类的构造函数
};
```
在这个示例中,Derived 类继承了 Base 类的构造函数。使用 using 关键字可以将基类的构造函数引入到派生类的作用域中,从而可以在派生类的构造函数中直接使用基类的构造函数。例如:
```c++
Derived d(1, 2); // 使用基类的构造函数初始化 d
```
这里使用了 Base 类的构造函数来初始化 Derived 类的对象 d。需要注意的是,继承构造函数只能用于构造函数的继承,不能用于其它函数的继承。此外,需要注意继承构造函数会继承基类的访问控制,因此需要保证基类的构造函数是 public 访问级别的。
相关问题
c++ 虚函数多继承
在C++中,虚函数多继承指的是一个类从多个基类继承,并且这些基类都有同名的虚函数。这种情况下,派生类需要处理虚函数的重复定义问题。
当一个派生类继承了多个基类,且这些基类都有同名的虚函数时,派生类需要使用作用域解析运算符来指定调用哪个基类的虚函数。具体来说,可以通过基类名加上作用域解析运算符来调用特定的虚函数。
例如,假设有两个基类 Base1 和 Base2,它们都有一个同名的虚函数 foo(),而派生类 Derived 继承了这两个基类。如果要在 Derived 类中调用 Base1 的 foo() 函数,可以使用 `Base1::foo()` 来指定调用 Base1 的虚函数。同样地,要调用 Base2 的 foo() 函数,则可以使用 `Base2::foo()`。
需要注意的是,虚函数多继承可能导致菱形继承问题(Diamond Inheritance),即多个基类继承同一个共同的基类。为了解决这个问题,可以使用虚继承(virtual inheritance)来避免重复继承共同基类。虚继承可以使得共同基类只有一个实例,从而避免重复定义和内存浪费。
总结起来,虚函数多继承是指派生类从多个基类继承,并且这些基类都有同名的虚函数。通过作用域解析运算符可以指定调用特定的虚函数。虚继承是解决菱形继承问题的方法之一。
解析以下概念:继承、抽象类、接口、多态、构造函数、static、final
1. 继承(Inheritance):一种面向对象编程的机制,允许一个类从另一个类继承属性和方法。继承的类被称为子类或派生类,被继承的类被称为父类或基类。子类可以重写父类的方法或属性,也可以新增自己的方法或属性。
2. 抽象类(Abstract Class):一种不能被实例化的类,只能作为超类被其他类继承。抽象类通常包含抽象方法或抽象属性,这些方法或属性只有声明,没有具体实现。子类必须实现它们才能被实例化。
3. 接口(Interface):一种定义了一组方法或属性的抽象类型。接口中的方法或属性没有具体实现,只有声明。类可以实现一个或多个接口,实现接口的类必须实现接口中所有的方法或属性。
4. 多态(Polymorphism):一种允许同一个方法在不同的对象上有不同行为的机制。多态可以通过继承、接口、重载等方式实现。它可以提高代码的复用性和灵活性。
5. 构造函数(Constructor):一种特殊的方法,用于创建对象时初始化对象的属性。构造函数的名称与类名相同,没有返回值。
6. static:一种关键字,用于修饰类的属性和方法。静态属性和方法属于类,而不属于对象。静态属性和方法可以通过类名直接访问。
7. final:一种关键字,用于修饰类、方法和变量。final修饰的类不能被继承,修饰的方法不能被重写,修饰的变量只能被赋值一次。
相关推荐
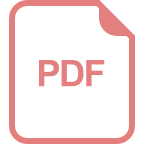












