设计程序,实现生产者/消费者进程(线程)的同步与互斥。在该程序中创建4个进程(或线程)模拟生产者和消费者,实现进程(线程)的同步与互斥。
时间: 2024-04-30 11:21:55 浏览: 16
以下是一个基于Python的生产者/消费者问题解决方案,使用了互斥锁和条件变量来实现进程的同步与互斥。
```python
import threading
import time
import random
# 创建互斥锁和条件变量
mutex = threading.Lock()
not_empty = threading.Condition(mutex)
not_full = threading.Condition(mutex)
# 缓冲区大小
buffer_size = 10
buffer = []
# 生产者函数
def producer():
global buffer
while True:
not_full.acquire() # 加锁
while len(buffer) == buffer_size:
not_full.wait() # 等待缓冲区不满
item = random.randint(0, 100) # 随机生成一个元素
buffer.append(item) # 将元素加入缓冲区
print("Producer produced:", item)
not_empty.notify() # 通知消费者
not_full.release() # 解锁
time.sleep(random.random()) # 随机等待一段时间
# 消费者函数
def consumer():
global buffer
while True:
not_empty.acquire() # 加锁
while len(buffer) == 0:
not_empty.wait() # 等待缓冲区不空
item = buffer.pop(0) # 取出缓冲区中的第一个元素
print("Consumer consumed:", item)
not_full.notify() # 通知生产者
not_empty.release() # 解锁
time.sleep(random.random()) # 随机等待一段时间
# 创建生产者和消费者线程
for i in range(2):
threading.Thread(target=producer).start()
for i in range(2):
threading.Thread(target=consumer).start()
```
在上面的代码中,我们首先创建了一个互斥锁和两个条件变量。然后,在生产者函数中,我们先使用 `not_full.acquire()` 加锁,然后检查缓冲区是否已满,如果满了就使用 `not_full.wait()` 将当前线程阻塞,直到消费者消费了一些元素后通知它继续生产。接着,我们生成一个随机元素并将其添加到缓冲区中,然后使用 `not_empty.notify()` 通知消费者。最后,我们使用 `not_full.release()` 解锁互斥锁并随机等待一段时间。
在消费者函数中,我们也先使用 `not_empty.acquire()` 加锁,然后检查缓冲区是否为空,如果是则使用 `not_empty.wait()` 将当前线程阻塞,直到生产者生产了一些元素后通知它继续消费。接着,我们从缓冲区中取出第一个元素并打印出来,然后使用 `not_full.notify()` 通知生产者。最后,我们使用 `not_empty.release()` 解锁互斥锁并随机等待一段时间。
最后,我们创建了两个生产者和两个消费者线程,并启动它们。这样,我们就可以模拟一个生产者/消费者问题,并通过互斥锁和条件变量实现了进程的同步与互斥。
相关推荐
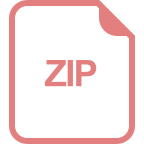
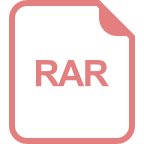
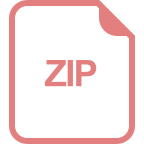














