在类里使用python opencv实现鼠标区域截图.
时间: 2024-05-11 12:16:17 浏览: 141
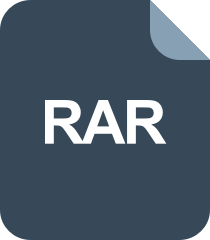
opencv实现截图功能

可以使用以下代码实现:
```python
import cv2
class MouseScreenshot:
def __init__(self):
self.drag_start = None
self.crop_rect = None
self.image = None
def mouse_handler(self, event, x, y, flags, param):
x, y = np.int16([x, y]) # fixed cv2.rectangle bug
if event == cv2.EVENT_LBUTTONDOWN:
self.drag_start = (x, y)
if event == cv2.EVENT_LBUTTONUP:
self.drag_start = None
if event == cv2.EVENT_MOUSEMOVE:
if self.drag_start:
x_min = min(x, self.drag_start[0])
y_min = min(y, self.drag_start[1])
x_max = max(x, self.drag_start[0])
y_max = max(y, self.drag_start[1])
self.crop_rect = (x_min, y_min, x_max, y_max)
self.draw_rect()
def crop_image(self):
if self.crop_rect:
self.image = self.image[self.crop_rect[1]:self.crop_rect[3],self.crop_rect[0]:self.crop_rect[2]]
cv2.imshow("cropped", self.image)
self.crop_rect = None
def draw_rect(self):
temp_image = np.copy(self.image)
if self.crop_rect:
cv2.rectangle(temp_image, (self.crop_rect[0], self.crop_rect[1]), (self.crop_rect[2], self.crop_rect[3]), (0, 255, 0), 2)
cv2.imshow("image", temp_image)
def run(self):
cv2.namedWindow("image")
cv2.setMouseCallback("image", self.mouse_handler)
while True:
cv2.imshow("image", self.image)
key = cv2.waitKey(1)
if key == ord("r"):
self.crop_rect = None
self.draw_rect()
elif key == ord("c"):
self.crop_image()
elif key == ord("q"):
cv2.destroyAllWindows()
return
elif key == ord("s"):
cv2.imwrite('screenshot.png',self.image)
def take_screenshot(self):
self.image = np.array(pyautogui.screenshot())
self.run()
```
你可以调用 `take_screenshot()` 方法来执行鼠标截图操作。当你点击鼠标左键并且拖动时,程序会显示一个矩形边界框,表明你想要截取的区域。你可以随时调整这个边界框,直到你满意为止。当你按下 `c` 键时,程序会截取并显示图片区域。你还可以按下 `s` 键将截屏保存到本地,或者按下 `q` 键退出程序。
阅读全文
相关推荐
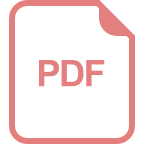
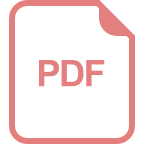




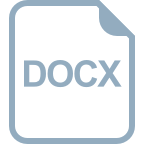
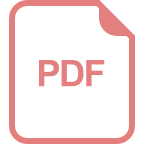
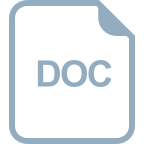
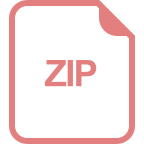
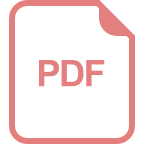
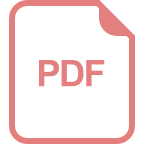



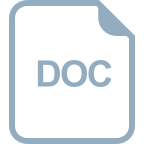

