surfaceView和相机预览
时间: 2023-09-08 16:10:00 浏览: 77
SurfaceView是一个可以在屏幕上绘制图像的窗口,而相机预览则是相机实时在屏幕上显示的图像。在使用相机预览时,可以使用SurfaceView作为相机预览界面,实现相机实时预览。在实现相机预览时,需要创建一个Camera对象,设置相机参数,然后将相机预览画面绑定到SurfaceView上,通过调用Camera对象的startPreview()方法,可以开始相机预览。在预览时,需要注意相机图像的宽高比与SurfaceView的宽高比是否一致,如果不一致需要进行适当的缩放。
相关问题
使用surface texture和surfaceview实现相机预览
好的,我会给您讲解使用 SurfaceTexture 和 SurfaceView 实现相机预览的方法。
首先,在布局文件中添加一个 SurfaceView:
```xml
<SurfaceView
android:id="@+id/surfaceView"
android:layout_width="match_parent"
android:layout_height="match_parent" />
```
然后在 Activity 或 Fragment 中获取 SurfaceView,并设置相机预览:
```java
// 获取 SurfaceView
SurfaceView surfaceView = findViewById(R.id.surfaceView);
// 获取相机实例
Camera camera = Camera.open();
try {
// 设置相机预览显示的 Surface
camera.setPreviewDisplay(surfaceView.getHolder());
// 开始预览
camera.startPreview();
} catch (IOException e) {
e.printStackTrace();
}
```
接下来,我们来使用 SurfaceTexture 来实现相机预览。
首先,在布局文件中添加一个 TextureView:
```xml
<TextureView
android:id="@+id/textureView"
android:layout_width="match_parent"
android:layout_height="match_parent" />
```
然后在 Activity 或 Fragment 中获取 TextureView,并设置相机预览:
```java
// 获取 TextureView
TextureView textureView = findViewById(R.id.textureView);
// 获取相机实例
Camera camera = Camera.open();
try {
// 创建 SurfaceTexture
SurfaceTexture surfaceTexture = new SurfaceTexture(0);
// 设置相机预览显示的 Surface
camera.setPreviewTexture(surfaceTexture);
// 开始预览
camera.startPreview();
} catch (IOException e) {
e.printStackTrace();
}
```
使用 SurfaceTexture 来实现相机预览的好处在于,可以通过 TextureView 的 setSurfaceTextureListener() 方法来监听 SurfaceTexture 的状态,例如当 SurfaceTexture 准备好后,可以获取它的宽高等信息,从而进行一些额外的操作。
希望这些代码可以帮助到您。
surfaceview 相机预览镜像
想要实现 SurfaceView 相机预览镜像,可以通过以下步骤实现:
1. 获取 Camera 对象,并设置预览回调函数。可以使用下面的代码实现:
```
Camera mCamera = Camera.open();
mCamera.setPreviewCallback(this);
```
2. 获取 SurfaceHolder 对象,并设置 SurfaceView 的宽高以及预览格式。可以使用下面的代码实现:
```
SurfaceHolder holder = getHolder();
holder.setFixedSize(width, height);
holder.setType(SurfaceHolder.SURFACE_TYPE_PUSH_BUFFERS);
```
3. 在预览回调函数中,将预览数据进行镜像翻转操作,然后将数据绘制到 SurfaceView 上。可以使用下面的代码实现:
```
@Override
public void onPreviewFrame(byte[] data, Camera camera) {
// 镜像翻转操作
int previewWidth = camera.getParameters().getPreviewSize().width;
int previewHeight = camera.getParameters().getPreviewSize().height;
int[] argb = ImageUtil.convertYUVtoARGB(data, previewWidth, previewHeight);
argb = ImageUtil.mirror(argb, previewWidth, previewHeight);
Bitmap bitmap = Bitmap.createBitmap(argb, previewWidth, previewHeight, Bitmap.Config.ARGB_8888);
// 绘制到 SurfaceView 上
Canvas canvas = getHolder().lockCanvas();
canvas.drawBitmap(bitmap, 0, 0, null);
getHolder().unlockCanvasAndPost(canvas);
}
```
其中,`ImageUtil.convertYUVtoARGB` 方法用于将 YUV 数据转换为 ARGB 数据,`ImageUtil.mirror` 方法用于对图像进行镜像翻转操作。
注意:上面的代码仅供参考,具体实现需要根据自己的需求进行调整。
阅读全文
相关推荐
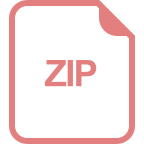
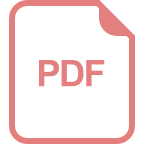
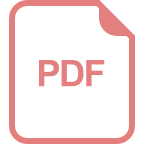













