C语言,用递归方法实现简单选择排序和冒泡排序
时间: 2023-11-23 21:02:20 浏览: 35
简单选择排序的递归实现:
```
void selectionSortRecursive(int arr[], int n, int index) {
// Base case
if (index == n-1)
return;
// Find the minimum element in unsorted array
int minIndex = index;
for (int i = index+1; i < n; i++)
if (arr[i] < arr[minIndex])
minIndex = i;
// Swap the found minimum element with the first element
int temp = arr[index];
arr[index] = arr[minIndex];
arr[minIndex] = temp;
// Recursive call for remaining unsorted array
selectionSortRecursive(arr, n, index+1);
}
```
冒泡排序的递归实现:
```
void bubbleSortRecursive(int arr[], int n) {
// Base case
if (n == 1)
return;
// One pass of bubble sort. After this pass, the largest element is moved (or bubbled) to end.
for (int i = 0; i < n-1; i++)
if (arr[i] > arr[i+1]) {
// Swap arr[i], arr[i+1]
int temp = arr[i];
arr[i] = arr[i+1];
arr[i+1] = temp;
}
// Recursive call for remaining array
bubbleSortRecursive(arr, n-1);
}
```
相关推荐
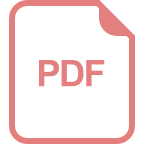
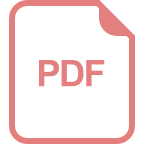
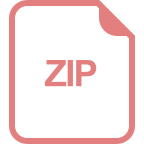
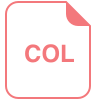
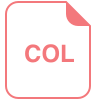
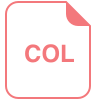
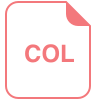
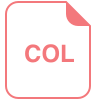









