Error deserializing key/value for partition dolphinscheduler-datalineage-task-param-0 at offset 0. If needed, please seek past the record to continue consumption.
时间: 2024-04-03 08:35:00 浏览: 18
这个错误是由于 Kafka 消费者在消费消息时无法反序列化消息所导致的。你需要检查消息生产者和消费者之间的序列化/反序列化方式是否相同,以及消息的格式是否正确。你可以尝试跳过当前消息,继续消费后续消息,或者删除当前消息并重新启动消费者。如果问题依然存在,可能需要查看 Kafka 的日志文件以获取更多信息。
相关问题
Kafka error deserializing key/value for partition可能是什么原因
Kafka error deserializing key/value for partition通常是因为消费者在尝试反序列化消息键或值时遇到了问题。有几个可能的原因:
1. 序列化和反序列化的方式不匹配:消息生产者使用一种序列化方式,而消费者使用另一种方式,导致反序列化失败。
2. 消息键或值格式错误:消息生产者可能意外更改了消息键或值的格式,导致消费者无法正确地反序列化。
3. 消息键或值为空:如果消息键或值为空,消费者尝试反序列化时可能会抛出异常。
4. 消息内容被破坏:在传输过程中,消息内容可能被损坏,导致消费者无法正确地反序列化。
为了解决这个问题,可以检查消息生产者和消费者使用的序列化方式是否相同,以及消息键或值的格式是否正确。此外,可以尝试使用Kafka自带的工具来验证消息内容是否正确。如果发现消息内容被破坏,可以考虑重新发送消息或使用更可靠的传输协议。
spring.jackson.date-format
spring.jackson.date-format is a configuration property in Spring Boot that allows you to specify the date format to be used when serializing and deserializing date/time values in JSON. By default, Spring uses the ISO-8601 format for date/time values, but you can customize the format using this property.
For example, if you want to use the format "yyyy-MM-dd HH:mm:ss.SSS", you can add the following line to your application.properties file:
```
spring.jackson.date-format=yyyy-MM-dd HH:mm:ss.SSS
```
This will tell Spring to use this format when serializing and deserializing date/time values.
相关推荐














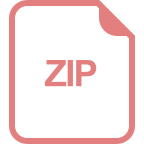
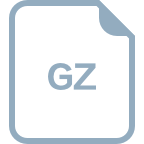
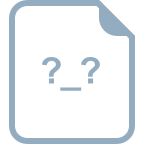