图书馆的书对应的数据类型定义如下: struct book { char name_of_book[100]; //书名 char author[25]; //作者 }; 编写函数实现:统计某一作者编写了几本书。 然后编写相应的主函数输入若干数据,并调用这个函数实现相应功能。 输入提示:"请输入书的数量:\n" 输入格式:%d 输入提示:"Please input %d name_of_book and author:\n" 输入格式:%s %s 输入提示:"请输入作者的姓名并统计他编写书的数量:\n" 输入格式:%s 输出格式:"作者%s编写了%d本书\n" 程序运行结果示例: 请输入书的数量: 5 Please input 1 name_of_book and author: c zhao Please input 2 name_of_book and author: java li Please input 3 name_of_book and author: c++ wang Please input 4 name_of_book and author: vb li Please input 5 name_of_book and author: vc li 请输入作者的姓名并统计他编写书的数量: li li作者编写了3本书
时间: 2023-12-10 22:37:36 浏览: 73
以下是代码实现:
```c
#include <stdio.h>
#include <string.h>
struct book {
char name_of_book[100];
char author[25];
};
int count_books(struct book books[], int n, char author[]) {
int count = 0;
for (int i = 0; i < n; i++) {
if (strcmp(books[i].author, author) == 0) {
count++;
}
}
return count;
}
int main() {
int n;
printf("请输入书的数量:\n");
scanf("%d", &n);
struct book books[n];
printf("Please input %d name_of_book and author:\n", n);
for (int i = 0; i < n; i++) {
scanf("%s %s", books[i].name_of_book, books[i].author);
}
char author[25];
printf("请输入作者的姓名并统计他编写书的数量:\n");
scanf("%s", author);
int count = count_books(books, n, author);
printf("作者%s编写了%d本书\n", author, count);
return 0;
}
```
程序运行示例:
```
请输入书的数量:
5
Please input 5 name_of_book and author:
c zhao
java li
c++ wang
vb li
vc li
请输入作者的姓名并统计他编写书的数量:
li
作者li编写了2本书
```
相关推荐
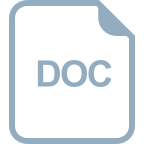
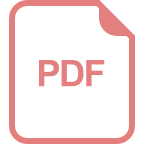
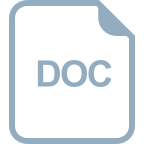
















