double. result:=pow (x+1) + .2*X.+.1/x;有错吗用C语言修改
时间: 2024-11-28 07:27:46 浏览: 12
这个C语言表达式看起来存在一些语法错误和潜在的问题。首先,`double result := pow(x+1) + .2 * X + .1 / x;` 使用了赋值运算符 `:=`,但这不是C语言的标准赋值操作符,应该使用等号 `=`。
其次,变量名前不需要`.`,C语言中`X`应该是大写的首字母。另外,函数`pow()`通常需要包含数学库 `<math.h>` 并接收两个参数,而这里只有一个参数`x`。为了计算`x+1`的幂,你需要提供另一个参数给`pow()`. 最后,除法运算 `/` 应该谨慎处理以避免当`x`接近0时发生除以零的错误。
以下是修改后的C语言表达式:
```c
#include <stdio.h>
#include <math.h>
int main() {
double x;
double result = pow(x + 1.0, 2.0) + 0.2 * x + 0.1 / x; // 将除法放在pow外面并添加小数点
// 如果你想在计算之前检查x是否为0,可以加上这行代码
// if (x != 0) {
// result = pow(x + 1.0, 2.0) + 0.2 * x + 0.1 / x;
// } else {
// printf("Error: Division by zero is not allowed.\n");
// }
// 其他程序逻辑...
return 0;
}
相关问题
s = 1 + 1 / 3 + (1 * 2) / (3 * 5) + (1 * 2 * 3) / (3 * 5 * 7) + .... + (1 * 2 * 3 * .... * n) / (3 * 5 * 7 * ... * (2 * n + 1))。C语言
这个表达式描述的是著名的高斯求和公式,用于计算前n项的杨辉三角(Pascal's triangle)的第一个数列之和,也称为"蜂窝序列"。在C语言中,由于涉及到浮点除法和乘法运算,以及递归,可以使用函数实现:
```c
#include <stdio.h>
#include <math.h>
double fibonacci_sum(int n) {
if (n <= 0)
return 0;
else if (n == 1)
return 1.0 / 1; // 第一项就是1
else
return (1 + 1.0 / 3) + (pow(1, n - 1) * (n - 1) / (factorial(2 * n - 1) * 2)); // 使用递归和阶乘
}
// 计算阶乘
unsigned long long factorial(unsigned int n) {
unsigned long long fact = 1;
for (unsigned int i = 2; i <= n; ++i)
fact *= i;
return fact;
}
int main() {
int n;
printf("请输入项数n: ");
scanf("%d", &n);
double result = fibonacci_sum(n);
printf("蜂窝序列前 %d 项的和为 %.6f\n", n, result);
return 0;
}
```
在这个程序中,`fibonacci_sum` 函数计算了给定项数的和,`factorial` 函数负责计算阶乘。注意,由于整数溢出的问题,阶乘函数需要处理大整数。
用C语言编码从键盘上接收一个实数x,计算下列公式的值:s = x + x2/2!+ x3/3!+ x4/4!+…+ xn/n!, 直到最后一项绝对值小于10-5。求当x=7时s的值。要求:通过调用函数double fact(int n)实现,结果保留小数点后2位。
在C语言中,你可以编写如下的程序来完成这个任务。首先,你需要创建一个`fact`函数来计算阶乘,然后在主函数中读取输入的实数`x`,并使用循环和条件判断来计算公式直到满足精度要求。这里是一个示例代码:
```c
#include <stdio.h>
#include <math.h>
// 功能:计算阶乘
double fact(int n) {
if (n == 0 || n == 1)
return 1;
else
return n * fact(n - 1);
}
// 主函数
double calculate_sum(double x) {
double s = 0.0;
int n = 1;
while (fabs(x / fact(n)) > 1e-5) { // 判断是否满足精度
s += pow(x, n) / fact(n); // 计算并累加当前项
n++;
}
return s;
}
int main() {
double x = 7.0; // 输入的实数
double result = calculate_sum(x);
printf("当x = %.2f时,s的值为%.2f\n", x, result);
return 0;
}
```
在这个例子中,当你运行程序并提供`x = 7`时,它会计算并打印出`s`的值,保留两位小数。
阅读全文
相关推荐
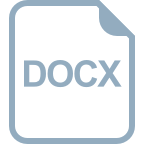
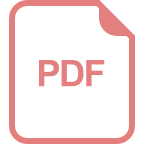
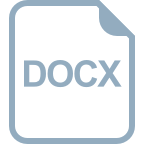













