main(int argc, char * argv[])实现拖拽图片进程序读图像的opencv代码
时间: 2024-09-23 18:06:29 浏览: 13
在OpenCV(计算机视觉库)中,`main(int argc, char* argv[])`是一个标准的C++程序入口点,用于接收命令行参数。如果你想让程序接受用户通过鼠标拖拽图片进来并读取,你需要做的是:
1. **包含必要的头文件**:
```cpp
#include <opencv2/opencv.hpp>
#include <opencv2/highgui/highgui.hpp>
```
2. **设置窗口模式**,并监听鼠标事件:
```cpp
cv::namedWindow("Drag and Drop", cv::WINDOW_NORMAL);
cv::setMouseCallback("Drag and Drop", mouse_callback_function, (void*)0);
```
这里 `mouse_callback_function` 是一个回调函数,会在鼠标按下、移动和释放时被调用。
3. **编写鼠标拖拽回调函数**:
```cpp
static void mouse_callback_function(int event, int x, int y, int flags, void* userdata) {
if (event == cv::EVENT_LBUTTONDOWN) {
// 记录下鼠标的起始位置
start_x = x;
start_y = y;
} else if (event == cv::EVENT_MOUSEMOVE && flags & cv::EVENT_FLAG_LBUTTON) {
// 当鼠标左键按住时,计算图片应该显示的位置
int dx = x - start_x;
int dy = y - start_y;
// 然后在这两个坐标处放置image ROI( Region Of Interest)
cv::imshow("Drag and Drop", src_roi(dx, dy, img.cols - dx, img.rows - dy));
}
}
```
4. **处理文件打开**:
当用户直接将文件拖到窗口上时,可以检查`argv`数组来获取图片路径:
```cpp
for (int i = 1; i < argc; ++i) {
std::string file_path = argv[i];
if (cv::imread(file_path)) {
Mat img = imread(file_path);
// ...然后将img传递给回调函数...
} else {
std::cout << "Failed to open image: " << file_path << std::endl;
}
}
```
5. **最后别忘了关闭窗口**:
```cpp
cv::waitKey(0);
cv::destroyAllWindows();
```
记得替换`src_roi`为你实际处理ROI的部分,并且这个例子假设你已经有了一个名为`img`的Mat变量保存了原始图片。
相关推荐
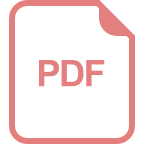
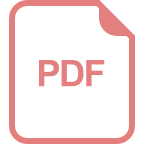










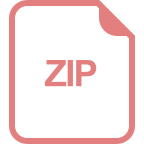
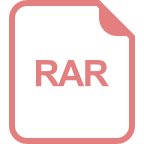
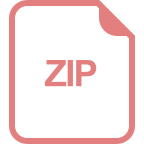
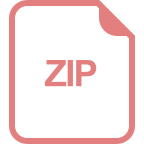
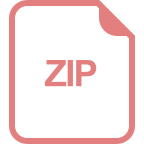