列表每一行记录一个学生姓名学号和五个成绩,输出所有学生姓名学号和最高的三次成绩并将结果保存在一个excel文件中,格式如下:学号姓名成绩1成绩2成绩3python代码
时间: 2023-12-16 17:04:21 浏览: 51
下面是一个可能的实现,需要使用 `pandas` 和 `openpyxl` 库:
```python
import pandas as pd
# 读取数据
df = pd.read_csv('students.csv', names=['name', 'id', 'score1', 'score2', 'score3', 'score4', 'score5'])
# 计算每个学生的最高三次成绩
df['top3'] = df[['score1', 'score2', 'score3', 'score4', 'score5']].apply(lambda x: sorted(x, reverse=True)[:3], axis=1)
# 拆分成三列
df[['topscore1', 'topscore2', 'topscore3']] = pd.DataFrame(df['top3'].tolist(), index=df.index)
# 只保留需要的列,并按学号排序
result = df[['id', 'name', 'topscore1', 'topscore2', 'topscore3']].sort_values(by='id')
# 保存到Excel文件
result.to_excel('result.xlsx', index=False)
```
说明:
1. 假设数据文件名为 `students.csv`,格式如下:
```
小明,1001,80,90,95,70,85
小红,1002,85,75,90,80,95
小刚,1003,95,85,80,90,75
...
```
2. `df` 是一个 `pandas` 的 `DataFrame` 对象,包含了所有学生的姓名、学号和五个成绩。
3. `df['top3']` 是一个新的列,其中每个元素是该学生的最高三次成绩,用一个列表表示。
4. `df[['topscore1', 'topscore2', 'topscore3']]` 创建了三个新的列,分别表示每个学生的最高成绩、次高成绩和第三高成绩。
5. `result` 是一个新的 `DataFrame` 对象,只包含需要输出的列,并按学号排序。
6. `result.to_excel('result.xlsx', index=False)` 将结果保存到 Excel 文件中,其中 `index=False` 表示不保存行索引。
相关推荐
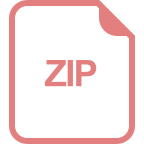
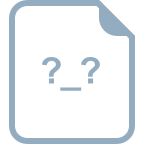
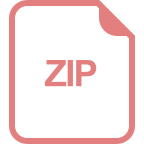














