normalize python
时间: 2023-08-31 21:10:22 浏览: 45
Normalizing in Python refers to the process of scaling the values of a variable to a standard range. This is done to make sure that all variables are on the same scale and to improve the performance of machine learning algorithms.
There are different methods for normalizing data in Python, such as:
1. Min-Max Scaling: This method scales the values to a range between 0 and 1. The formula for min-max scaling is:
normalized_value = (value - min_value) / (max_value - min_value)
2. Z-Score Normalization: This method scales the values to have a mean of 0 and a standard deviation of 1. The formula for z-score normalization is:
normalized_value = (value - mean) / standard_deviation
3. Decimal Scaling: This method scales the values by multiplying them by a power of 10. The power of 10 is determined by the maximum absolute value of the variable. The formula for decimal scaling is:
normalized_value = value / 10^k
where k is the number of digits required to represent the maximum absolute value of the variable.
Here is an example of how to normalize data using the Min-Max Scaling method in Python:
```python
from sklearn.preprocessing import MinMaxScaler
import numpy as np
# create sample data
data = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
# create scaler object
scaler = MinMaxScaler()
# fit and transform data
normalized_data = scaler.fit_transform(data)
print(normalized_data)
```
Output:
```
[[0. 0. 0. ]
[0.5 0.5 0.5]
[1. 1. 1. ]]
```
In this example, the MinMaxScaler object is used to normalize the data. The fit_transform method is called on the scaler object to both fit the scaler to the data and transform the data. The resulting normalized data is printed to the console.
相关推荐
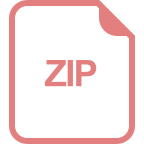
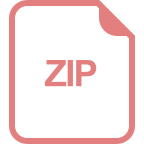














