编写主函数测试以下程序派生类构造函数执行的顺序,并分析其原因。 #include<iostream> using namespace std; class B1 { public: B1(int i) { cout << "constructing B1" << i << endl; } ~B1(){ cout << "destructing B1" << endl; } }; class B2 { public: B2(int i) { cout << "constructing B2" << i << endl; } ~B2() { cout << "destructing B2" << endl; } }; class B3 { public: B3() { cout << "constructing B3 *"<< endl; } ~B3() { cout << "destructing B3" << endl; } }; class C :public B2, public B1, public B3 { public: C(int a,int b,int c,int d):B1(a),memberB2(d),memberB1(c),B2(b){} private: B1 memberB1; B2 memberB2; B3 memberB3; }; int main() { }
时间: 2024-03-18 10:45:08 浏览: 118
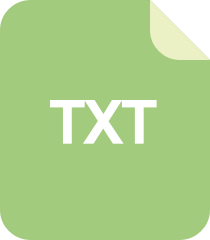
#include_iostream.h_

可以先分析一下类C的继承结构和成员变量。类C是由B2、B1、B3三个类派生而来的,而且还有三个成员变量memberB1、memberB2、memberB3。其中,memberB1和memberB2是B1和B2类的对象,而memberB3是B3类的对象。
在构造函数中,我们可以看到B1和B2类的构造函数是在C类构造函数的初始化列表中直接构造的,而B3类的构造函数是在C类的构造函数中进行构造的。而memberB1和memberB2则是由默认构造函数构造的,因为没有在初始化列表中进行初始化。
所以,程序运行时,先构造B1类,然后构造B2类,接着构造B3类,最后构造memberB1、memberB2和memberB3。析构函数的执行顺序与构造函数的执行顺序相反,先析构memberB3、memberB2和memberB1,然后析构B3类,接着析构B2类,最后析构B1类。
当然,在main函数中需要实例化一个C类对象,并且需要传入4个参数,这样才能触发构造函数的执行。
阅读全文
相关推荐
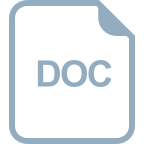
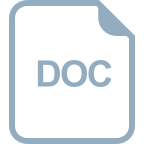





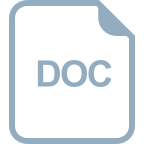
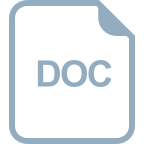



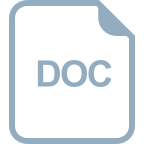
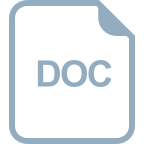
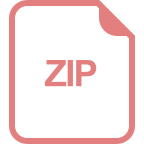
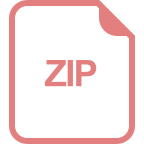