unity 写一个协程对象池管理的脚本
时间: 2024-03-14 07:48:27 浏览: 21
好,以下是一个简单的协程对象池管理的脚本,使用C#编写:
```csharp
using System.Collections;
using System.Collections.Generic;
using UnityEngine;
public class CoroutineObjectPool : MonoBehaviour
{
public GameObject prefab;
public int poolSize = 10;
private List<GameObject> objectPool = new List<GameObject>();
private void Start()
{
for (int i = 0; i < poolSize; i++)
{
GameObject newObj = Instantiate(prefab, transform);
newObj.SetActive(false);
objectPool.Add(newObj);
}
}
public IEnumerator SpawnObjectFromPool(Vector3 position, Quaternion rotation, float delay)
{
GameObject obj = GetObjectFromPool();
obj.transform.position = position;
obj.transform.rotation = rotation;
obj.SetActive(true);
yield return new WaitForSeconds(delay);
obj.SetActive(false);
}
private GameObject GetObjectFromPool()
{
for (int i = 0; i < objectPool.Count; i++)
{
if (!objectPool[i].activeInHierarchy)
{
return objectPool[i];
}
}
GameObject newObj = Instantiate(prefab, transform);
newObj.SetActive(false);
objectPool.Add(newObj);
return newObj;
}
}
```
使用方法:
1. 将该脚本挂载到一个GameObject上;
2. 在Inspector面板中设置要管理的对象的预制体(prefab)和对象池大小(poolSize);
3. 在代码中调用SpawnObjectFromPool方法即可从对象池中获取对象并激活,经过指定的延迟后再将其隐藏回收。
示例代码:
```csharp
public class SpawnObject : MonoBehaviour
{
public CoroutineObjectPool poolManager;
public float delay = 2f;
private void Start()
{
StartCoroutine(Spawn());
}
private IEnumerator Spawn()
{
while (true)
{
yield return new WaitForSeconds(delay);
Vector3 position = transform.position;
Quaternion rotation = transform.rotation;
StartCoroutine(poolManager.SpawnObjectFromPool(position, rotation, delay));
}
}
}
```
在上述示例代码中,每隔指定的时间间隔,从SpawnObject的位置和旋转角度获取一个对象,并经过指定的延迟时间后将其回收。
相关推荐
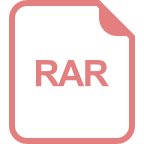
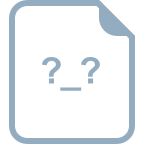
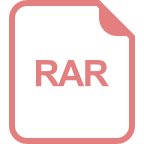
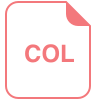













