stm32按键控制LED的流水显示方向。
时间: 2023-10-19 18:15:16 浏览: 33
以下是一个基本的代码示例,可以通过按键控制LED的流水显示方向:
```c
#include "stm32f10x.h"
#define LED_GPIO GPIOB
#define LED_GPIO_CLK RCC_APB2Periph_GPIOB
#define KEY_GPIO GPIOA
#define KEY_GPIO_CLK RCC_APB2Periph_GPIOA
#define KEY_PIN GPIO_Pin_0
#define LED_PIN GPIO_Pin_12
void LED_Init(void) {
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(LED_GPIO_CLK, ENABLE);
GPIO_InitStructure.GPIO_Pin = LED_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(LED_GPIO, &GPIO_InitStructure);
}
void KEY_Init(void) {
GPIO_InitTypeDef GPIO_InitStructure;
RCC_APB2PeriphClockCmd(KEY_GPIO_CLK, ENABLE);
GPIO_InitStructure.GPIO_Pin = KEY_PIN;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPU;
GPIO_Init(KEY_GPIO, &GPIO_InitStructure);
}
int main(void) {
LED_Init();
KEY_Init();
uint8_t led_state = 0; // 初始灯的状态为0
uint8_t direction = 1; // 初始流水方向为正向
while (1) {
// 检测按键状态
if (GPIO_ReadInputDataBit(KEY_GPIO, KEY_PIN) == 0) {
// 按键按下,改变流水方向
direction = -direction;
while (GPIO_ReadInputDataBit(KEY_GPIO, KEY_PIN) == 0) {} // 等待按键释放
}
// 更新LED状态
led_state += direction;
if (led_state < 0) {
led_state = 9;
} else if (led_state > 9) {
led_state = 0;
}
LED_GPIO->ODR = (uint16_t)(1 << led_state);
// 延时一段时间
for (int i = 0; i < 100000; i++) {}
}
}
```
代码中使用PA0作为按键输入,PB12作为LED输出,每按下一次按键就会改变LED流水的方向。具体实现方法是使用一个变量来记录当前LED状态,然后根据流水方向不断更新LED状态并延时一段时间,使得LED以流水的形式显示。
相关推荐
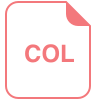
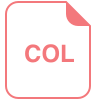
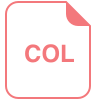
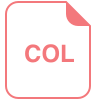
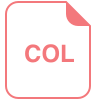









