python如何读取tfrecord文件_Python如何读取tfrecord文件使用pytorch读取tfrecord文件而不依赖tensordflow,python,PyTorch,TensorD...
时间: 2023-07-18 12:35:08 浏览: 65
Python读取TFRecord文件的方法如下:
```python
import tensorflow as tf
# 创建一个TFRecordDataset对象
dataset = tf.data.TFRecordDataset('data.tfrecord')
# 定义读取函数
def parser(record):
features = {
'image': tf.io.FixedLenFeature([], dtype=tf.string),
'label': tf.io.FixedLenFeature([], dtype=tf.int64)
}
parsed = tf.io.parse_single_example(record, features)
image = tf.io.decode_jpeg(parsed['image'], channels=3)
label = parsed['label']
return image, label
# 应用读取函数到每个record
dataset = dataset.map(parser)
# 创建迭代器
iterator = dataset.make_one_shot_iterator()
# 获取数据
image, label = iterator.get_next()
```
以上代码演示了如何读取名为`data.tfrecord`的TFRecord文件,并解析其中的图像和标签信息。在解析函数`parser`中,我们先定义了TFRecord文件中包含的特征信息,然后使用`tf.io.parse_single_example`函数解析单个record,并对图像数据进行解码。最后,我们使用`map`函数将解析函数应用到每个record上。
当然,如果您使用的是PyTorch,也可以使用以下代码读取TFRecord文件:
```python
import torch
import torchvision.datasets as datasets
import torchvision.transforms as transforms
# 定义解析函数
def parser(record):
features = {
'image': tf.io.FixedLenFeature([], dtype=tf.string),
'label': tf.io.FixedLenFeature([], dtype=tf.int64)
}
parsed = tf.io.parse_single_example(record, features)
image = tf.io.decode_jpeg(parsed['image'], channels=3)
label = parsed['label']
return image, label
# 创建数据集对象
dataset = datasets.DatasetFolder(
'data.tfrecord',
loader=lambda x: torch.load(x),
extensions=('tfrecord')
)
# 应用解析函数到每个record
dataset.transform = transforms.Compose([
parser
])
# 创建数据加载器
dataloader = torch.utils.data.DataLoader(
dataset,
batch_size=32,
shuffle=True
)
# 获取数据
for images, labels in dataloader:
# 使用数据进行训练或预测
pass
```
以上代码演示了如何使用PyTorch的`DatasetFolder`读取TFRecord文件,并使用解析函数`parser`解析图像和标签信息。最后,我们创建了一个数据加载器,并使用其中的数据进行训练或预测。
相关推荐
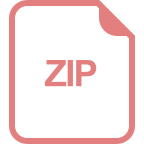
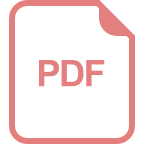
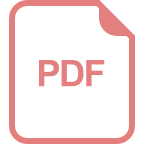














