给出python代码实现,通过处理图像的频域数据,使得反傅里叶变换后的图像旋转角度会发生相应的变化。具体来说,如果对频域数据进行旋转,反傅里叶变换后的图像也会相应地旋转。这是由于傅里叶变换与反傅里叶变换是对称的,因此频域数据的变化必然会对图像的空间域数据产生影响
时间: 2024-05-14 12:15:50 浏览: 44
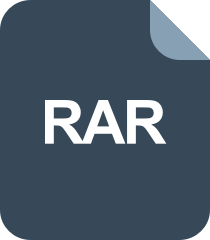
图像傅里叶变换

。很高兴回答你的问题!为了实现你所提供的功能,可以执行以下步骤来处理频域数据并旋转反傅里叶变换后的图像:
步骤1:使用NumPy和OpenCV库读取图像并进行灰度化处理。
```python
import cv2
import numpy as np
# Load the image
img = cv2.imread("image.jpg")
# Convert the image to grayscale
gray_img = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
```
步骤2:对图像的灰度像素值进行傅里叶变换以获取频域数据。
```python
# Perform a 2D Fast Fourier Transform on the image
f = np.fft.fft2(gray_img)
# Shift the zero-frequency component to the center of the spectrum
fshift = np.fft.fftshift(f)
# Compute the magnitude spectrum of the frequency components
magnitude_spectrum = 20*np.log(np.abs(fshift))
```
步骤3:将频域数据进行旋转,并计算反傅里叶变换。
```python
# Determine the angle of rotation
angle = 30 # example angle
# Rotate the frequency components by the desired angle
rows, cols = gray_img.shape
cx, cy = int(cols/2), int(rows/2) # Find the center of the image
rotation_matrix = cv2.getRotationMatrix2D((cx,cy), angle, 1) # Create a rotation matrix
rotated_fshift = cv2.warpAffine(fshift, rotation_matrix, (cols, rows)) # Rotate the frequency components
# Shift the zero-frequency component back to the top-left corner
rotated_f = np.fft.ifftshift(rotated_fshift)
# Compute the inverse Fourier Transform to obtain the rotated image
rotated_img = np.abs(np.fft.ifft2(rotated_f))
```
通过上述步骤,可以通过处理图像的频域数据实现反傅里叶变换后的图像旋转角度相应地变化。希望这能对你有所帮助!
阅读全文
相关推荐
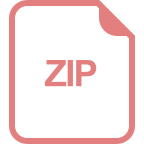
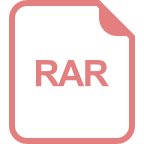
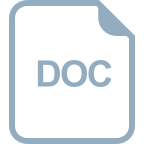
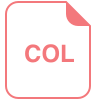
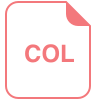
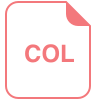
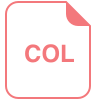
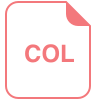
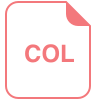
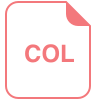







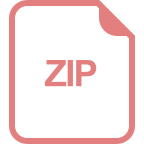