optics聚类算法 python
时间: 2023-10-12 12:21:23 浏览: 132
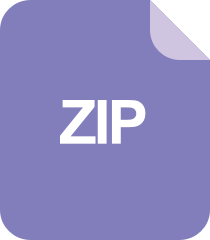
基于Python实现聚类算法【100011024】
Optics 聚类算法是一种基于密度的聚类算法,它可以处理任意形状的簇,并且不需要预设簇的数量。在 Python 中,可以使用 Scikit-learn 库来实现 Optics 聚类算法。具体实现步骤包括数据预处理、构建 Optics 对象、拟合数据和可视化聚类结果等。以下是一个简单的示例代码:
```python
from sklearn.cluster import OPTICS
# 准备数据
X = [[2, 4], [2, 5], [4, 6], [4, 7], [4, 8], [6, 9], [6, 10], [7, 8]]
# 构建 Optics 对象
optics_model = OPTICS(min_samples=2, xi=0.05)
# 拟合数据
optics_model.fit(X)
# 可视化聚类结果
import matplotlib.pyplot as plt
labels = optics_model.labels_
reachability = optics_model.reachability_
plt.figure(figsize=(10, 7))
plt.title('Optics Clustering')
plt.scatter(X[:,0], X[:,1], c=labels, cmap='viridis')
plt.show()
```
该示例代码使用了一个简单的二维数据集,构建 Optics 对象时设置了最小样本数为 2,$\xi$ 参数为 0.05。最后,可视化聚类结果并展示给用户。
阅读全文
相关推荐
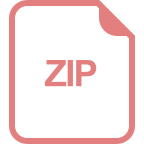






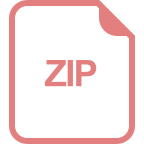
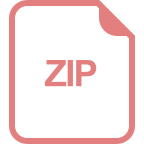
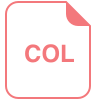

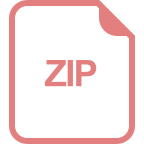
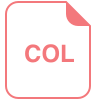
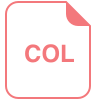
