Warning Color Space is set to one of the ACES options, but OCIO ACES has not been set up. Read the tooltip of Set Up OCIO ACES and decide whether to set up ACES or switch Color Space to "Linear" or "Gamma 2.2".
时间: 2024-04-27 20:19:50 浏览: 8
这似乎是一个关于色彩空间的警告信息,提示你当前选择的色彩空间是ACES之一,但是OCIO ACES没有被设置。你需要阅读设置OCIO ACES的工具提示,并决定是设置ACES还是切换色彩空间为“Linear”或“Gamma 2.2”。具体的操作步骤可能因软件或工具而异,你可以查找相应的文档或向相关的技术支持人员咨询。
相关问题
Write me a java project named pontoon game, which needs to meet the following requirements:Pontoon is a card game played with three decks of playing cards (i.e. cards of 2-10, Jack, Queen, King, and Ace from the four suits of Hearts, Diamonds, Spades, and Clubs). There are two players: the user of the computer, and the computer itself. The object of the game is to beat the other player by either getting as close to a score of 21 as possible (without exceeding 21) or by having 5 cards and a score of 21 or less. Each card has a value: numeric cards have their numeric value, 'face' cards have value 10, and aces have value 11. The game is played as follows, Firstly the user is told about the game and invited to play. If they opt to play then four cards are dealt. The first card goes to the user, the second to the computer, the third to the user, and the fourth to the computer. The computer's card remains hidden, all others are shown. The user can then indicate they wish to have an additional card ("hit"). If they are this card is also displayed. Additional cards may be taken until either the user's score exceeds 21 (they "bust"), or they choose not to take another card ("sit"/"stand"). Once the user has finished taking cards the computer will take cards. The computer will always take a card if their total is less than 16 (and the user's score doesn't exceed 21).
Sure, here is an implementation of the Pontoon game in Java:
```
import java.util.ArrayList;
import java.util.Collections;
import java.util.List;
import java.util.Scanner;
public class PontoonGame {
private static final int MAX_SCORE = 21;
private static final int MAX_NUM_CARDS = 5;
private static final int DEALER_STAND_THRESHOLD = 16;
private static final int CARD_VALUE_ACE = 11;
private static final int CARD_VALUE_FACE = 10;
private static final String[] SUITS = new String[] { "Hearts", "Diamonds", "Spades", "Clubs" };
private static final String[] RANKS = new String[] { "2", "3", "4", "5", "6", "7", "8", "9", "10", "Jack", "Queen", "King", "Ace" };
private List<Card> deck;
private List<Card> playerHand;
private List<Card> dealerHand;
public PontoonGame() {
deck = new ArrayList<>();
playerHand = new ArrayList<>();
dealerHand = new ArrayList<>();
// Initialize deck
for (String suit : SUITS) {
for (String rank : RANKS) {
deck.add(new Card(rank, suit));
}
}
}
public void play() {
Scanner scanner = new Scanner(System.in);
System.out.println("Welcome to Pontoon! Would you like to play? (y/n)");
String input = scanner.nextLine();
if (!input.equalsIgnoreCase("y")) {
return;
}
// Shuffle deck and deal cards
Collections.shuffle(deck);
playerHand.add(dealCard());
dealerHand.add(dealCard());
playerHand.add(dealCard());
dealerHand.add(dealCard());
// Show initial hands
System.out.println("Your hand: " + playerHand);
System.out.println("Dealer's hand: " + dealerHand.get(0) + ", [hidden]");
// Player's turn
while (true) {
System.out.println("Would you like to hit or stand? (h/s)");
input = scanner.nextLine();
if (input.equalsIgnoreCase("h")) {
playerHand.add(dealCard());
System.out.println("Your hand: " + playerHand);
if (getHandValue(playerHand) > MAX_SCORE) {
System.out.println("Bust! You lose.");
return;
}
} else if (input.equalsIgnoreCase("s")) {
break;
} else {
System.out.println("Invalid input. Please enter 'h' or 's'.");
}
}
// Dealer's turn
while (getHandValue(dealerHand) < DEALER_STAND_THRESHOLD && getHandValue(playerHand) <= MAX_SCORE) {
dealerHand.add(dealCard());
}
System.out.println("Dealer's hand: " + dealerHand);
// Determine winner
int playerScore = getHandValue(playerHand);
int dealerScore = getHandValue(dealerHand);
if (playerScore > MAX_SCORE) {
System.out.println("Bust! You lose.");
} else if (dealerScore > MAX_SCORE) {
System.out.println("Dealer busts! You win.");
} else if (playerScore == dealerScore) {
System.out.println("It's a tie!");
} else if (playerScore == MAX_SCORE || dealerScore > MAX_SCORE || playerScore > dealerScore) {
System.out.println("You win!");
} else {
System.out.println("Dealer wins!");
}
}
private Card dealCard() {
if (deck.isEmpty()) {
throw new IllegalStateException("Deck is empty.");
}
return deck.remove(0);
}
private int getHandValue(List<Card> hand) {
int value = 0;
int numAces = 0;
for (Card card : hand) {
if (card.getRank().equals("Ace")) {
numAces++;
} else if (card.getRank().equals("Jack") || card.getRank().equals("Queen") || card.getRank().equals("King")) {
value += CARD_VALUE_FACE;
} else {
value += Integer.parseInt(card.getRank());
}
}
for (int i = 0; i < numAces; i++) {
if (value + CARD_VALUE_ACE > MAX_SCORE) {
value += 1;
} else {
value += CARD_VALUE_ACE;
}
}
return value;
}
private static class Card {
private final String rank;
private final String suit;
public Card(String rank, String suit) {
this.rank = rank;
this.suit = suit;
}
public String getRank() {
return rank;
}
public String getSuit() {
return suit;
}
@Override
public String toString() {
return rank + " of " + suit;
}
}
public static void main(String[] args) {
PontoonGame game = new PontoonGame();
game.play();
}
}
```
This implementation uses object-oriented programming principles to model the game as a `PontoonGame` class that contains methods for playing the game. The game logic is implemented in the `play()` method, which prompts the user for input, deals cards, and determines the winner. The `Card` class is a nested class that represents a playing card with a rank and a suit.
tonemapping aces
ACES(Academy Color Encoding System)是由美国学术电影艺术科学院开发的一种高动态范围(HDR)图像色彩编码系统。其目的是提供一种标准化的方法,用于在不同设备和平台之间进行色彩一致性和交互性。
ACES的一个重要组成部分是Tonemapping技术。Tonemapping是一种将高动态范围图像转换为标准动态范围(SDR)图像的过程,以便在普通显示设备上进行正确显示。ACES Tonemapping使用一种非线性算法,以保持原始图像的亮度和色彩细节,同时确保图像在SDR设备上显示出适当的亮度和对比度。
ACES Tonemapping的主要目标是在不失真地映射高动态范围数据的同时,提供适当的辐射度和对比度。它通过分析输入图像的高动态范围信息,然后应用适当的压缩和曲线变化来实现这一目标。这种算法的目的是在可视范围内保留细节,并在亮度过渡区域中提供更平滑的过渡。
ACES Tonemapping算法的应用可以在电影和电视制作,游戏开发以及摄影等领域中看到。它是一种标准化的方法,可以确保在不同设备和平台上展示的图像保持色彩一致性和艺术家意图的准确性。
总而言之,ACES Tonemapping是一种将高动态范围图像转换为标准动态范围图像的技术,旨在保持亮度和色彩细节的同时,在SDR设备上提供适当的亮度和对比度。它是ACES系统的一个重要组成部分,用于在不同设备和平台上实现色彩一致性和艺术家意图的准确展示。
相关推荐
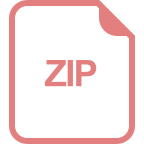
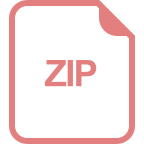












