在这里给出函数被调用进行测试的例子。例如: #include <stdio.h> #include<stdlib.h> struct node {int ch; struct node * next;}; struct node *setlink(int N); //建链表函数,已经定义 int findnode(struct node * head);//在下边代码区定义此函数 int main() { int N; struct node *head; scanf("%d",&N); head=setlink(N); printf("%d", findnode(head)); return 0; } /* 请在这里填写答案 */
时间: 2024-03-03 21:50:33 浏览: 112
好的,下面是一个简单的实现,用于在链表中查找某个值的位置:
```c++
#include <stdio.h>
#include <stdlib.h>
struct node {
int ch;
struct node *next;
};
struct node *setlink(int N) {
struct node *head = NULL, *tail = NULL;
for (int i = 0; i < N; i++) {
int ch;
scanf("%d", &ch);
struct node *p = (struct node *)malloc(sizeof(struct node));
p->ch = ch;
p->next = NULL;
if (head == NULL) {
head = tail = p;
} else {
tail->next = p;
tail = p;
}
}
return head;
}
int findnode(struct node *head) {
int ch;
scanf("%d", &ch);
struct node *p = head;
int pos = 1;
while (p != NULL) {
if (p->ch == ch) {
return pos;
}
p = p->next;
pos++;
}
return -1;
}
int main() {
int N;
struct node *head;
scanf("%d", &N);
head = setlink(N);
printf("%d", findnode(head));
return 0;
}
```
在这个程序中,我们首先定义了一个结构体 `node`,用于表示链表节点。在 `setlink()` 函数中,我们根据输入的数据建立了一个链表,并返回链表头节点的指针。在 `findnode()` 函数中,我们首先读入需要查找的值 `ch`,然后遍历链表,查找是否存在该值。如果找到了,我们返回该节点的位置;否则,返回 `-1`。在 `main()` 函数中,我们首先读入需要建立的链表的长度 `N`,然后调用 `setlink()` 函数来建立链表,并将链表头节点的指针赋给变量 `head`。最后,我们调用 `findnode()` 函数来查找链表中的某个节点,输出其位置。
相关推荐
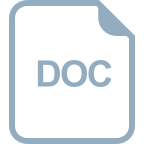
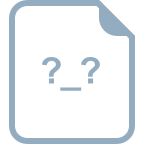
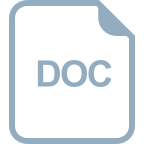











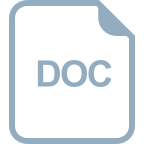
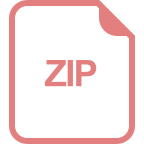
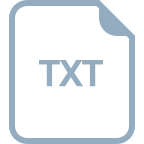
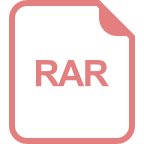