# 加载原始图像和压缩后的图像 original_image = Image.open('0.jpg') compressed_image = Image.open('1.jpg')
时间: 2023-12-08 19:03:04 浏览: 123
好的,你的第一个问题是如何加载原始图像和压缩后的图像,对吧?那么这个问题的答案就是使用Python的PIL库中的Image模块来加载图像。具体的代码如下:
```python
from PIL import Image
# 加载原始图像
original_image = Image.open('0.jpg')
# 加载压缩后的图像
compressed_image = Image.open('1.jpg')
```
其中,`'0.jpg'`和`'1.jpg'`分别为原始图像和压缩后的图像的文件路径。注意,这里假设你已经安装了PIL库,如果没有安装需要先安装。
相关问题
from PIL import Image import numpy as np import io # 读取原始图像和压缩后图像 original_img = Image.open('test.jpg') compressed_img = Image.open('test_compressed.jpg') # 将图像转换为 NumPy 数组 original_img_arr = np.array(original_img) compressed_img_arr = np.array(compressed_img) # 计算原始图像大小 original_size = original_img_arr.nbytes # 计算压缩后图像大小 compressed_size = compressed_img_arr.nbytes # 计算压缩率 compression_ratio = compressed_size / original_size # 计算峰值信噪比(PSNR) mse = np.mean((original_img_arr - compressed_img_arr) ** 2) psnr = 10 * np.log10(255**2 / mse) # 计算结构相似性指数(SSIM) from skimage.metrics import structural_similarity as ssim ssim_score = ssim(original_img_arr, compressed_img_arr, multichannel=True) # 计算峰值信噪比改进比(PSNR-HVS) from skimage.metrics import peak_signal_noise_ratio as psnr_hvs psnr_hvs_score = psnr_hvs(original_img_arr, compressed_img_arr, data_range=original_img_arr.max()) # 计算多样性信噪比(MS-SSIM) from skimage.metrics import multi_scale_ssim as ms_ssim ms_ssim_score = ms_ssim(original_img_arr, compressed_img_arr, data_range=original_img_arr.max(), win_size=11) # 计算复杂度压缩比(CPC) cpc = psnr / compression_ratio # 输出七种压缩率 print(f"Compression ratio: {compression_ratio:.4f}") print(f"Peak Signal-to-Noise Ratio (PSNR): {psnr:.2f}") print(f"Structural Similarity Index (SSIM): {ssim_score:.4f}") print(f"Peak Signal-to-Noise Ratio - HVS (PSNR-HVS): {psnr_hvs_score:.2f}") print(f"Multi-Scale Structural Similarity (MS-SSIM): {ms_ssim_score:.4f}") print(f"Complexity-Compression Ratio (CPC): {cpc:.2f}") print(f"Original size: {original_size:,}") print(f"Compressed size: {compressed_size:,}")ValueError: operands could not be broadcast together with shapes (417,556,3) (418,558,3)
这个错误通常是由于两个数组的形状不匹配导致的。在您的情况下,您正在尝试将形状为(417,556,3)和(418,558,3)的两个数组进行广播操作,但它们的形状不兼容。
根据您提供的代码,错误可能出现在以下几行中:
```python
original_img_arr = np.array(original_img)
compressed_img_arr = np.array(compressed_img)
```
这两行代码将 PIL.Image 对象转换为 NumPy 数组。如果两个图像的大小不同,则它们的数组形状也不同,这可能导致广播错误。
您可以尝试查看这两张图像的大小,看看它们是否匹配。或者,您可以尝试调整图像的大小,使它们具有相同的形状,然后再将它们转换为 NumPy 数组。
例如,您可以使用 PIL 库中的 resize() 函数来调整图像的大小:
```python
original_img = original_img.resize((width, height))
compressed_img = compressed_img.resize((width, height))
```
其中,width 和 height 是您想要的新图像的宽度和高度。然后,您可以将这两张图像转换为 NumPy 数组,并继续计算 PSNR、SSIM 等指标。
import numpy as np from sklearn.cluster import KMeans def compress_image(image, n_clusters): # 转换为一维向量 data = image.reshape(-1, 3) # 用 KMeans 算法将像素点聚类到 n_clusters 个簇中 kmeans = KMeans(n_clusters=5, random_state=0).fit(data) # 将每个像素点替换为所属簇的中心像素值 compressed_data = np.array([kmeans.cluster_centers_[label] for label in kmeans.labels_]) # 将压缩后的一维向量转换回原图像的形状 compressed_image = compressed_data.reshape(image.shape) return compressed_imageimport matplotlib.pyplot as plt from PIL import Image # 读取图像 image = np.array(Image.open('0.jpg')) # 将图像数据归一化到 [0, 1] 范围内 image = image.astype('float32') / 255.0 # 压缩图像 compressed_image = compress_image(image_norm, n_clusters=16) # 显示压缩前后的图像 fig, ax = plt.subplots(1, 2, figsize=(10, 5)) ax[0].imshow(image) ax[0].set_title('Original Image') ax[1].imshow(compressed_image) ax[1].set_title('Compressed Image') plt.show()加上计算压缩率的功能
可以在 compress_image 函数中添加计算压缩率的功能,具体实现如下:
```
import numpy as np
from sklearn.cluster import KMeans
def compress_image(image, n_clusters):
# 转换为一维向量
data = image.reshape(-1, 3)
# 用 KMeans 算法将像素点聚类到 n_clusters 个簇中
kmeans = KMeans(n_clusters=n_clusters, random_state=0).fit(data)
# 将每个像素点替换为所属簇的中心像素值
compressed_data = np.array([kmeans.cluster_centers_[label] for label in kmeans.labels_])
# 将压缩后的一维向量转换回原图像的形状
compressed_image = compressed_data.reshape(image.shape)
# 计算压缩率
compressed_size = compressed_data.nbytes
original_size = image.nbytes
compression_ratio = compressed_size / original_size
return compressed_image, compression_ratio
import matplotlib.pyplot as plt
from PIL import Image
# 读取图像
image = np.array(Image.open('0.jpg'))
# 将图像数据归一化到 [0, 1] 范围内
image = image.astype('float32') / 255.0
# 压缩图像
n_clusters = 5
compressed_image, compression_ratio = compress_image(image, n_clusters)
# 显示压缩前后的图像
fig, ax = plt.subplots(1, 2, figsize=(10, 5))
ax[0].imshow(image)
ax[0].set_title('Original Image')
ax[1].imshow(compressed_image)
ax[1].set_title('Compressed Image')
# 显示压缩率
plt.figtext(0.5, 0.9, f'Compression Ratio: {compression_ratio:.2%}', ha='center', fontsize=12)
plt.show()
```
在上述代码中,计算压缩率的代码为:
```
compressed_size = compressed_data.nbytes
original_size = image.nbytes
compression_ratio = compressed_size / original_size
```
其中,`compressed_data.nbytes` 表示压缩后的图像数据的字节数,`image.nbytes` 表示原始图像数据的字节数,压缩率即为两者之比。
阅读全文
相关推荐




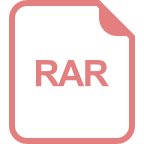
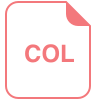
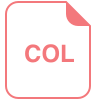






