c语言多文件编写单链表
时间: 2024-09-13 19:02:54 浏览: 43
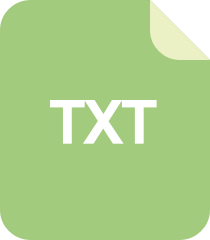
c语言:创建单链表的头插法代码
在C语言中,使用多文件编写单链表可以将代码结构分解,提高代码的组织性和可维护性。下面是基本步骤:
1. **声明头文件** (list.h):
```c
#ifndef LIST_H
#define LIST_H
struct Node {
int data;
struct Node* next;
};
// 定义链表的基本操作,如创建节点、插入节点等
void create_node(struct Node** head);
void insert_node(struct Node** head, int value);
#endif
```
2. **定义函数体** (list.c):
```c
#include "list.h"
void create_node(struct Node** head) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
if (newNode != NULL) {
newNode->data = 0; // 初始化数据
newNode->next = *head;
*head = newNode;
} else {
printf("Memory allocation failed.\n");
}
}
void insert_node(struct Node** head, int value) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
if (newNode != NULL) {
newNode->data = value;
newNode->next = (*head)->next;
(*head)->next = newNode;
} else {
printf("Memory allocation failed.\n");
}
}
```
3. **主程序** (main.c) 或其他源文件中引用并使用:
```c
#include "list.h"
int main() {
struct Node* head = NULL;
create_node(&head); // 创建头结点
// 插入节点到链表...
insert_node(&head, 1);
insert_node(&head, 2);
// ...
return 0;
}
```
阅读全文
相关推荐
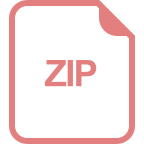
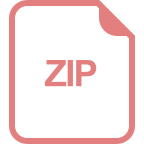















