创建一棵二叉树,计算二叉树结点个数、叶子结点个数、二叉树深度、复制二叉树并将其以前序遍历、中序遍历、后序遍历进行输出
时间: 2024-12-13 13:15:29 浏览: 8
创建一棵二叉树通常涉及节点结构的定义,每个节点包含两个指针(指向左子节点和右子节点),以及一些数据元素。以下是基本步骤:
1. **创建节点**:
```python
class TreeNode:
def __init__(self, val=0, left=None, right=None):
self.val = val
self.left = left
self.right = right
```
2. **计算节点个数**:
```python
def count_nodes(root):
if root is None:
return 0
else:
return 1 + count_nodes(root.left) + count_nodes(root.right)
```
3. **计算叶子节点个数**(没有子节点的节点):
```python
def count_leaves(root):
if root is None:
return 0
elif root.left is None and root.right is None:
return 1
else:
return count_leaves(root.left) + count_leaves(root.right)
```
4. **计算深度(最远叶节点的距离)**:
```python
def max_depth(root):
if root is None:
return 0
else:
left_depth = max_depth(root.left)
right_depth = max_depth(root.right)
return max(left_depth, right_depth) + 1
```
5. **复制二叉树**:
```python
def clone_tree(root):
if root is None:
return None
cloned = TreeNode(root.val)
cloned.left = clone_tree(root.left)
cloned.right = clone_tree(root.right)
return cloned
```
6. **遍历**:
- **前序遍历**: (根节点 -> 左子树 -> 右子树)
- **中序遍历**: (左子树 -> 根节点 -> 右子树)
- **后序遍历**: (左子树 -> 右子树 -> 根节点)
对于遍历,你可以分别编写递归函数,例如 `preorder_traversal`, `inorder_traversal`, 和 `postorder_traversal`。
阅读全文
相关推荐
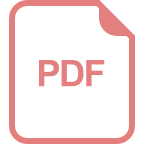
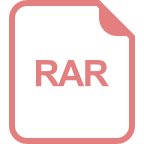















