static u8 key=1; if(mode)key=1;//连续扫描按键 if(key==1&&(KEY1==0||KEY2==0||KEY3==0||KEY4==0))解释一下这段51单片机代码
时间: 2024-10-14 09:12:27 浏览: 68
这段代码是在51单片机这样的嵌入式系统中常见的,使用了静态存储区(static)变量 `u8 key=1;` 来表示一个按键的状态。`u8` 表示无符号8位整型,`key` 初始值为1,通常表示按键未按下。
下面是整个代码段的解释:
1. `static u8 key=1;`: 宣告了一个名为 `key` 的静态变量,其类型为无符号8位整数,初始化为1,代表按键默认处于未按下状态。
2. `if(mode)key=1;`: 如果条件 `mode` 为真,这里会将 `key` 重置为1,可能是为了对按键进行连续扫描。在这种情况下,`mode` 可能是一个触发按键扫描的标志信号。
3. `if(key==1&&(KEY1==0||KEY2==0||KEY3==0||KEY4==0))`: 进行另一个判断,如果 `key` 仍为1(即按键未释放),同时任一 `KEY1`, `KEY2`, `KEY3`, 或 `KEY4` 键没有被按下(这些通常是外接的输入引脚,值为0表示按下),则该部分代码块内的内容将会执行。这可能意味着当某个键被按下的时候,需要执行特定的操作。
简而言之,这段代码用于监控一组按键,只有在连续按键并且所有指定的外部按键都没有被按下时才会执行后续操作。
相关问题
完善以下代码 u8 KEY_Scan(u8 mode) { static u8 key_up=1;//按键按松开标志 if(mode)key_up=1; //支持连按 if(key_up&&(KEY0==0||KEY1==0||WK_UP==1)) { delay_ms(10);//去抖动 key_up=0; if(KEY0==0)return KEY0_PRES; else if(KEY1==0)return KEY1_PRES; else if(WK_UP==1)return WKUP_PRES; }else if(KEY0==1&&KEY1==1&&WK_UP==0)key_up=1; return 0;// 无按键按下 }
这段代码看起来是针对单片机中的按键扫描函数。以下是一些可以改进的建议:
1. 函数名:函数名可以更加规范,建议使用大写字母和下划线的命名方式,如:KEY_SCAN。
2. 参数mode:参数mode没有明确的说明,可以添加注释或者修改参数名,以表明该参数的作用。
3. 函数返回值:函数返回值可以使用枚举类型,可以更加清晰地表达按键的状态。
4. 按键扫描:建议添加按键消抖功能,以避免按键不稳定的现象。
下面是改进后的代码:
```c
typedef enum {
KEY_NONE = 0, // 无按键按下
KEY0_PRES, // KEY0按下
KEY1_PRES, // KEY1按下
WKUP_PRES // WK_UP按下
} KEY_Status;
/**
* @brief 按键扫描函数
* @param mode 0:支持单次按键功能,1:支持连续按键功能
* @return KEY_Status 按键状态
*/
KEY_Status KEY_SCAN(u8 mode) {
static u8 key_up = 1; // 按键按松开标志
if (mode) key_up = 1; // 支持连按
if (key_up && (KEY0 == 0 || KEY1 == 0 || WK_UP == 1)) {
delay_ms(10); // 去抖动
key_up = 0;
if (KEY0 == 0) return KEY0_PRES;
else if (KEY1 == 0) return KEY1_PRES;
else if (WK_UP == 1) return WKUP_PRES;
} else if (KEY0 == 1 && KEY1 == 1 && WK_UP == 0) {
key_up = 1;
}
return KEY_NONE;
}
```
改进后的代码对函数名、参数、返回值等进行了规范化,同时添加了按键消抖功能,提高了按键扫描的稳定性。
u8 KEY_Scan(u8 mode) { static u8 key_up=1; if(mode)key_up=1; if(key_up&&(KEY0==0||KEY1==0||WK_UP==1)) { delay_ms(10); key_up=0; if(KEY0==0)return KEY0_PRES; else if(KEY1==0)return KEY1_PRES; else if(WK_UP==1)return WKUP_PRES; }else if(KEY0==1&&KEY1==1&&WK_UP==0)key_up=1; return 0;/ }
) { //检测按键是否按下 if(KEY0==0||KEY1==0||KEY2==0||WK_UP==1) { //延时消抖 delay_ms(10); key_up=0; if(KEY0==0)return KEY0_PRES; else if(KEY1==0)return KEY1_PRES; else if(KEY2==0)return KEY2_PRES; else if(WK_UP==1)return WKUP_PRES; } } else { //检测按键是否松开 if(KEY0==1&&KEY1==1&&KEY2==1&&WK_UP==0)key_up=1; } return 0;// 无按键按下}
Explanation:
This code is for detecting keypad input. It takes in a parameter 'mode', which is used to indicate if the program is in initialization mode (mode = 1). The program contains a debounce mechanism to avoid false inputs caused by the user pressing the key multiple times.
The function first checks if the key has been released (key_up == 1). If so, it checks if any key (KEY0, KEY1, KEY2, or WK_UP) has been pressed. If a key has been pressed, the program waits for 10 ms to debounce, and then returns the corresponding signal indicating which key has been pressed (KEY0_PRES, KEY1_PRES, KEY2_PRES, or WKUP_PRES).
If no key is pressed, the program checks if the key has been released, and sets key_up to 1 if it has. It then returns 0 to indicate no key has been pressed.
阅读全文
相关推荐
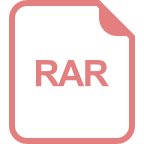
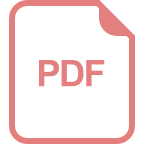
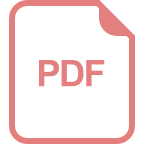













