需求:定义一个集合,用于存储3个学生对象 学生类的属性为:name,age,gender 定义一个工具类,用于获取集合中最大学生的年龄
时间: 2024-09-24 14:13:41 浏览: 10
为了实现这个需求,你可以按照以下步骤设计:
1. 定义`Student`类,包含姓名(name)、年龄(age)和性别(gender)三个属性。
```java
public class Student {
private String name;
private int age;
private String gender;
// 构造函数
public Student(String name, int age, String gender) {
this.name = name;
this.age = age;
this.gender = gender;
}
// 获取和设置属性的方法
// ...
}
```
2. 创建一个`CollectionsUtil`工具类,添加一个静态方法`findOldestStudent(AssociativeArray<Student> students)`,接收一个`Map<String, Student>`类型的集合,其中键是学生的姓名,值是学生对象。在这个方法中遍历集合,找到年龄最大的学生并返回。
```java
import java.util.Map;
public class CollectionsUtil {
public static Student findOldestStudent(Map<String, Student> studentCollection) {
if (studentCollection.isEmpty()) {
return null; // 如果集合为空,返回null
}
Student oldestStudent = studentCollection.values().iterator().next(); // 先取第一个作为初始假设
for (Student student : studentCollection.values()) {
if (student.getAge() > oldestStudent.getAge()) {
oldestStudent = student;
}
}
return oldestStudent;
}
}
```
现在你可以创建一个`Map`来存储学生,并使用`CollectionsUtil.findOldestStudent()`方法找出最年长的学生。
```java
Map<String, Student> studentList = new HashMap<>();
studentList.put("Alice", new Student("Alice", 20, "Female"));
studentList.put("Bob", new Student("Bob", 25, "Male"));
studentList.put("Charlie", new Student("Charlie", 22, "Male"));
Student oldestStudent = CollectionsUtil.findOldestStudent(studentList);
System.out.println("The oldest student is " + oldestStudent.getName() + ", with an age of " + oldestStudent.getAge());
```
相关推荐
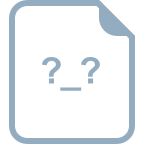
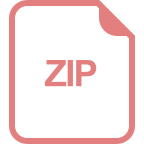
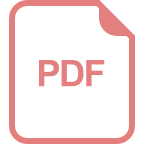














