用Python语言的OpenCV写一个png图片透明处理的代码
时间: 2023-08-31 22:25:54 浏览: 53
### 回答1:
可以使用以下代码来实现png图片透明处理:
import cv2
img = cv2.imread('image.png', cv2.IMREAD_UNCHANGED)
ret, mask = cv2.threshold(img[:,:,3], 10, 255, cv2.THRESH_BINARY)
mask_inv = cv2.bitwise_not(mask)
img_bg = cv2.bitwise_and(img[:,:,0:3], img[:,:,0:3], mask=mask_inv)
img_fg = cv2.bitwise_and(img[:,:,3], img[:,:,3], mask=mask)
dst = cv2.add(img_bg, img_fg)
cv2.imwrite('image_new.png', dst)
### 回答2:
要使用Python语言的OpenCV实现png图片透明处理,你可以按照以下步骤编写代码:
首先,导入必要的库:`opencv-python`和`numpy`。
```python
import cv2
import numpy as np
```
然后,使用`cv2.imread()`函数读取png图片,并将它存储为一个变量。
```python
image = cv2.imread('image.png', cv2.IMREAD_UNCHANGED)
```
接下来,判断图片是否具有alpha通道(即透明度通道)。如果没有,你可以选择将其添加到图片中:
```python
if image.shape[2] == 3: # 没有alpha通道
image = cv2.cvtColor(image, cv2.COLOR_BGR2BGRA) # 将图片转换为BGRA格式
```
然后,将图片中的所有像素的alpha通道值设置为0,以实现完全透明的效果。
```python
image[:, :, 3] = 0 # 设置所有像素的alpha值为0
```
最后,使用`cv2.imwrite()`函数将处理后的图片保存为另一个文件。
```python
cv2.imwrite('image_transparent.png', image)
```
完整的代码如下所示:
```python
import cv2
import numpy as np
image = cv2.imread('image.png', cv2.IMREAD_UNCHANGED)
if image.shape[2] == 3:
image = cv2.cvtColor(image, cv2.COLOR_BGR2BGRA)
image[:, :, 3] = 0
cv2.imwrite('image_transparent.png', image)
```
以上代码将读取名为`image.png`的png图片,将其处理成完全透明的图片,并保存为`image_transparent.png`。
### 回答3:
使用Python语言的OpenCV库,可以很方便地实现PNG图片的透明处理。下面是一个简单的代码示例:
```python
import cv2
# 读取PNG图片
image = cv2.imread('input.png', cv2.IMREAD_UNCHANGED)
# 获取图像的alpha通道
alpha_channel = image[:, :, 3]
# 将alpha通道设置为255,即完全不透明
alpha_channel[alpha_channel > 0] = 255
# 将修改后的alpha通道赋值回原图像
image[:, :, 3] = alpha_channel
# 保存处理后的图像
cv2.imwrite('output.png', image)
```
上述代码首先使用`cv2.imread()`函数读取PNG图片,并使用参数`cv2.IMREAD_UNCHANGED`来保持图像的原始通道信息。然后,通过索引操作获取图像的alpha通道,即透明度信息。
接下来,将alpha通道中不为0的像素点设置为255,即将其设置为完全不透明。这样做可以确保处理后的图片在任何显示设备上都可以正常显示。
最后,将修改后的alpha通道赋值回原图像,并使用`cv2.imwrite()`函数保存处理后的图像为PNG格式。
相关推荐
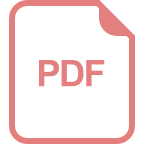
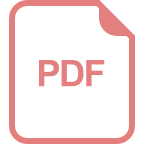
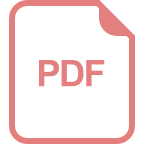














