用C++实现一个计算器程序,该计算器支持以下功能: (1)支持加、减、乘、除四则运算 (2)支持带括号的优先级运算
时间: 2024-10-20 14:05:32 浏览: 44
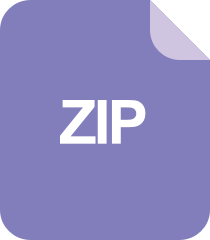
C++支持的四则运算计算器

为了创建一个C++计算器程序,我们可以使用递归解析器或者栈来处理表达式。这里是一个简单的命令行计算器示例,使用了栈数据结构来实现:
```cpp
#include <iostream>
#include <stack>
#include <cctype>
class Calculator {
public:
double calculate(const std::string &expression) {
std::stack<double> operands;
std::stack<char> operators;
for (char c : expression) {
if (isdigit(c)) {
// 如果遇到数字,直接压入堆栈
operands.push(c - '0');
} else if (c == '+' || c == '-' || c == '*' || c == '/') {
// 如果遇到操作符,取出最近的两个操作数并计算
while (!operators.empty() && hasPrecedence(operators.top(), c)) {
double b = operands.top(); operands.pop();
double a = operands.top(); operands.pop();
char op = operators.top(); operators.pop();
switch (op) {
case '+': operands.push(a + b); break;
case '-': operands.push(a - b); break;
case '*': operands.push(a * b); break;
case '/': operands.push(a / b); break;
}
}
operators.push(c);
} else if (c == '(') {
operators.push(c);
} else if (c == ')') {
while (!operators.top() != '(') {
double b = operands.top(); operands.pop();
double a = operands.top(); operands.pop();
char op = operators.top(); operators.pop();
switch (op) {
case '+': operands.push(a + b); break;
case '-': operands.push(a - b); break;
case '*': operands.push(a * b); break;
case '/': operands.push(a / b); break;
}
}
operators.pop(); // 出去左括号
}
}
// 处理剩余的操作符
while (!operators.empty()) {
double b = operands.top(); operands.pop();
double a = operands.top(); operands.pop();
char op = operators.top(); operators.pop();
switch (op) {
case '+': operands.push(a + b); break;
case '-': operands.push(a - b); break;
case '*': operands.push(a * b); break;
case '/': operands.push(a / b); break;
}
}
return operands.top(); // 返回最终结果
}
private:
bool hasPrecedence(char op1, char op2) {
// 简单的优先级判断,可以按实际需要调整
return (op2 == '*' || op2 == '/') && (op1 != '*' && op1 != '/');
}
};
int main() {
Calculator calc;
std::string input;
std::cout << "请输入一个数学表达式(如:5+3*(4-2)/2): ";
std::getline(std::cin, input);
try {
double result = calc.calculate(input);
std::cout << "计算结果: " << result << std::endl;
} catch (...) {
std::cerr << "输入的表达式有误!" << std::endl;
}
return 0;
}
```
在这个例子中,用户可以在命令行输入一个包含加减乘除和括号的表达式,程序会计算其结果。注意这是一个基础版本,对于更复杂的表达式解析和错误处理可能需要进一步优化。
阅读全文
相关推荐
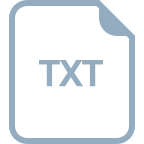
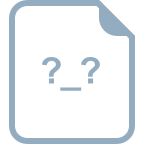


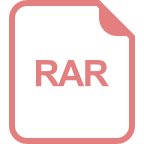
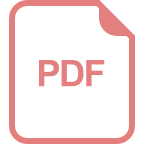
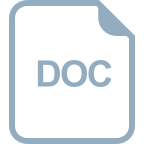
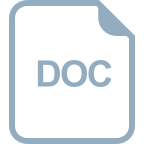
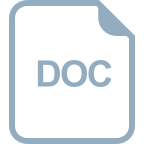
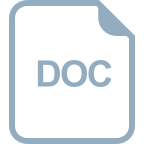
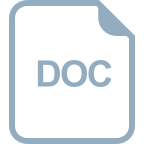
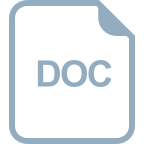





