写一个STM32的4*4矩阵键盘程序
时间: 2023-07-25 19:03:43 浏览: 118
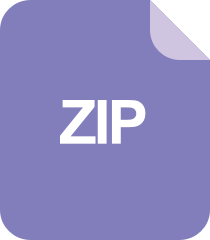
基于STM32的基于行扫描法的4*4矩阵键盘的实现代码
好的,以下是一个简单的基于STM32的4*4矩阵键盘程序:
```c
#include "stm32f10x.h"
GPIO_InitTypeDef GPIO_InitStructure;
void Delay(__IO uint32_t nCount) {
while(nCount--);
}
void GPIO_Configuration(void) {
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOA, ENABLE);
RCC_APB2PeriphClockCmd(RCC_APB2Periph_GPIOB, ENABLE);
// 设置矩阵键盘的行引脚为输出模式
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_0 | GPIO_Pin_1 | GPIO_Pin_2 | GPIO_Pin_3;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_Out_PP;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOA, &GPIO_InitStructure);
// 设置矩阵键盘的列引脚为输入模式
GPIO_InitStructure.GPIO_Pin = GPIO_Pin_4 | GPIO_Pin_5 | GPIO_Pin_6 | GPIO_Pin_7;
GPIO_InitStructure.GPIO_Mode = GPIO_Mode_IPD;
GPIO_InitStructure.GPIO_Speed = GPIO_Speed_50MHz;
GPIO_Init(GPIOB, &GPIO_InitStructure);
}
int main(void) {
uint8_t key[4][4] = { // 键码表
{1, 2, 3, 'A'},
{4, 5, 6, 'B'},
{7, 8, 9, 'C'},
{'*', 0, '#', 'D'}
};
uint8_t row, col;
uint8_t key_val = 0;
GPIO_Configuration();
while(1) {
for(row = 0; row < 4; row++) { // 遍历行
GPIO_SetBits(GPIOA, GPIO_Pin_0 << row);
for(col = 0; col < 4; col++) { // 遍历列
if(GPIO_ReadInputDataBit(GPIOB, GPIO_Pin_4 << col) == Bit_RESET) {
key_val = key[row][col]; // 获取键码
while(GPIO_ReadInputDataBit(GPIOB, GPIO_Pin_4 << col) == Bit_RESET); // 等待按键松开
}
}
GPIO_ResetBits(GPIOA, GPIO_Pin_0 << row);
}
if(key_val != 0) { // 检测到有按键按下
// TODO: 处理按键事件
key_val = 0;
}
Delay(1000); // 延时1ms
}
}
```
以上程序简单实现了4*4矩阵键盘的按键检测功能,当检测到按键按下时,会获取对应的键码,并在松开按键后进行处理。注意,该程序还需要根据实际需求进行进一步的功能扩展和优化。
阅读全文
相关推荐
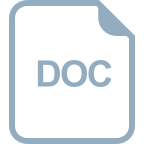
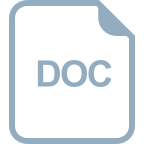
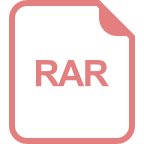
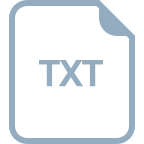
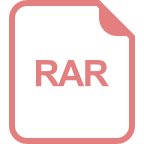

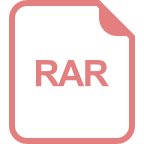
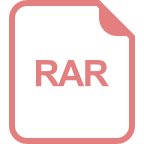
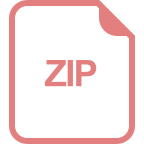
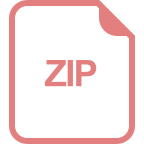
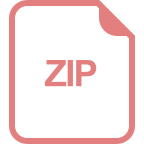
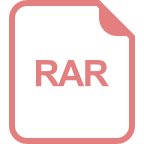
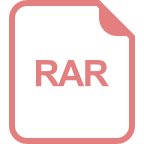
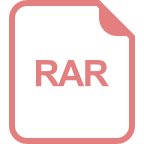



