给定一个 二又树的先序序列ABDEGCHF,中序序列DBEGAHCF,要求输出二叉树的后续序列 char * getPost(char preA[] , char inA[] , int N)
时间: 2024-03-23 08:37:43 浏览: 12
二叉树的后序遍历顺序为 左子树后序序列 + 右子树后序序列 + 根节点。
我们可以通过先序序列和中序序列构建二叉树,然后对二叉树进行后序遍历,即可得到后序序列。
首先,我们需要找到根节点在中序序列中的位置,然后可以得到左子树的中序序列和右子树的中序序列。接着在先序序列中,根节点后面的连续若干个节点是左子树的先序序列,剩下的是右子树的先序序列。
重复上述步骤,递归构建左子树和右子树,直到叶子节点为止,最后对整个树进行后序遍历,即可得到后序序列。
以下是代码实现:
```c++
char* getPost(char preA[], char inA[], int N) {
if (N == 0) return NULL;
char root = preA[0];
int pos = 0;
while (inA[pos] != root) pos++;
int leftSize = pos, rightSize = N - pos - 1;
char* leftPost = getPost(preA + 1, inA, leftSize);
char* rightPost = getPost(preA + 1 + leftSize, inA + leftSize + 1, rightSize);
char* postA = new char[N + 1];
if (leftPost) {
strcpy(postA, leftPost);
delete[] leftPost;
}
if (rightPost) {
strcat(postA, rightPost);
delete[] rightPost;
}
postA[N - 1] = root;
postA[N] = '\0';
return postA;
}
```
其中,N表示节点的个数,preA表示先序序列,inA表示中序序列,getPost函数返回后序序列。
测试代码如下:
```c++
int main() {
char preA[] = "ABDEGCHF";
char inA[] = "DBEGAHCF";
char* postA = getPost(preA, inA, strlen(preA));
cout << postA << endl; // 输出 GEBDHFCA
delete[] postA;
return 0;
}
```
相关推荐
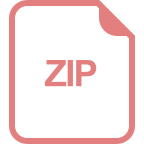
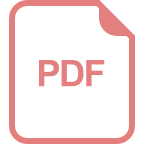
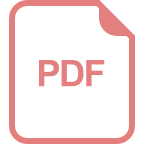














