dropout层怎么加入pytorch
时间: 2023-04-20 21:02:30 浏览: 140
在PyTorch中,可以使用nn.Dropout()函数来添加dropout层。具体操作如下:
1. 导入PyTorch库
```python
import torch
import torch.nn as nn
```
2. 定义模型
```python
class MyModel(nn.Module):
def __init__(self):
super(MyModel, self).__init__()
self.fc1 = nn.Linear(10, 20)
self.dropout = nn.Dropout(p=.5)
self.fc2 = nn.Linear(20, 2)
def forward(self, x):
x = self.fc1(x)
x = self.dropout(x)
x = self.fc2(x)
return x
```
在这个模型中,我们定义了一个包含两个全连接层和一个dropout层的神经网络。其中,dropout层的概率为.5。
3. 使用模型
```python
model = MyModel()
input_data = torch.randn(1, 10)
output = model(input_data)
```
在使用模型时,我们可以像上面这样输入数据,并得到输出结果。在这个过程中,dropout层会随机地将一些神经元的输出置为,以减少过拟合的风险。
相关问题
dropout层在pytorch
中的实现方式是什么?
在PyTorch中,可以使用`torch.nn.Dropout()`函数来实现Dropout层。该函数的语法如下:
```python
torch.nn.Dropout(p=0.5, inplace=False)
```
其中,`p`参数表示每个元素被置为0的概率,默认为0.5;`inplace`参数表示是否进行原地操作,即是否覆盖原来的输入张量,默认为False。
在模型中使用Dropout层时,只需将其作为模型的一层进行添加即可。例如,下面是一个使用Dropout的简单全连接神经网络模型:
```python
import torch.nn as nn
class Net(nn.Module):
def __init__(self):
super(Net, self).__init__()
self.fc1 = nn.Linear(784, 512)
self.dropout = nn.Dropout(p=0.5) # 添加Dropout层
self.fc2 = nn.Linear(512, 10)
def forward(self, x):
x = x.view(-1, 784)
x = self.fc1(x)
x = nn.functional.relu(x)
x = self.dropout(x) # 在模型中使用Dropout层
x = self.fc2(x)
return x
```
在上面的示例中,`self.dropout`表示添加了一个Dropout层,`x = self.dropout(x)`表示在模型中使用了Dropout层。
pytorch dropout层
PyTorch中的Dropout层是一种正则化技术,用于减少神经网络的过拟合现象。Dropout层在训练过程中随机地将一部分神经元的输出置为0,以一定的概率(通常为0.5)来减少神经元之间的依赖关系,从而提高模型的泛化能力。
在PyTorch中,可以通过torch.nn.Dropout类来实现Dropout层。具体使用方法如下:
```python
import torch
import torch.nn as nn
# 创建一个具有Dropout层的神经网络
class Net(nn.Module):
def __init__(self):
super(Net, self).__init__()
self.fc1 = nn.Linear(10, 20)
self.dropout = nn.Dropout(p=0.5)
self.fc2 = nn.Linear(20, 2)
def forward(self, x):
x = self.fc1(x)
x = self.dropout(x)
x = self.fc2(x)
return x
# 实例化网络
net = Net()
# 使用Dropout层进行前向传播
input_data = torch.randn(32, 10) # 输入数据
output = net(input_data) # 前向传播
```
在上面的例子中,我们创建了一个具有Dropout层的神经网络。在网络的初始化过程中,我们使用了nn.Dropout(p=0.5)来定义了一个Dropout层,其中p表示神经元被置为0的概率。在网络的前向传播过程中,我们调用dropout方法对输入进行处理,得到输出结果。
注意,在模型的训练过程中,需要使用net.train()来启用Dropout层,而在模型的测试过程中,需要使用net.eval()来关闭Dropout层。这是因为在训练过程中,Dropout层会随机地将部分神经元置为0,而在测试过程中,我们希望保留所有的神经元输出,以评估模型的性能。
阅读全文
相关推荐
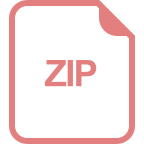
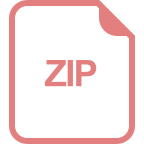
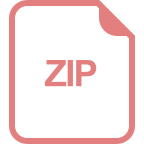
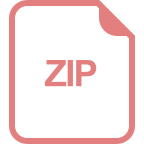
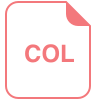











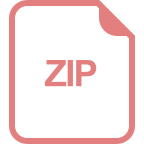