将模型转移到GPU上运行
时间: 2023-12-25 08:03:27 浏览: 99
要将模型转移到GPU上运行,需要确保你的计算机有可用的GPU并且已经安装了相应的CUDA工具包和深度学习框架。
以下是一个将模型转移到GPU上运行的基本步骤:
1. 检查GPU是否可用:在Python中使用`torch.cuda.is_available()`函数检查CUDA是否可用。
2. 将模型转移到GPU上:使用`model.cuda()`函数将模型转移到GPU上。
3. 将数据转移到GPU上:使用`data.cuda()`函数将数据转移到GPU上。
4. 将模型参数和优化器转移到GPU上:使用`model.to(device)`和`optimizer.to(device)`将模型和优化器转移到GPU上。
5. 在训练过程中使用GPU:在训练迭代过程中,将数据和模型的输入和输出转移到GPU上。
6. 在测试过程中使用GPU:在测试过程中,将数据和模型的输入和输出转移到GPU上。
以下是一个示例代码,将模型转移到GPU上运行:
```
import torch
import torch.nn as nn
import torch.optim as optim
# 定义模型
class MyModel(nn.Module):
def __init__(self):
super(MyModel, self).__init__()
self.fc1 = nn.Linear(10, 5)
self.fc2 = nn.Linear(5, 1)
def forward(self, x):
x = torch.relu(self.fc1(x))
x = self.fc2(x)
return x
# 检查CUDA是否可用
device = torch.device("cuda" if torch.cuda.is_available() else "cpu")
# 初始化模型和优化器
model = MyModel().to(device)
optimizer = optim.SGD(model.parameters(), lr=0.01)
# 将数据转移到GPU上
inputs = torch.randn(5, 10).to(device)
labels = torch.randn(5, 1).to(device)
# 训练模型
for epoch in range(10):
optimizer.zero_grad()
outputs = model(inputs)
loss = nn.functional.mse_loss(outputs, labels)
loss.backward()
optimizer.step()
print("Epoch [{}/{}], Loss: {:.4f}".format(epoch+1, 10, loss.item()))
# 测试模型
test_inputs = torch.randn(2, 10).to(device)
with torch.no_grad():
test_outputs = model(test_inputs)
print("Test Outputs:", test_outputs)
```
阅读全文
相关推荐
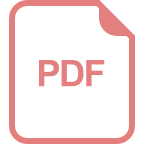



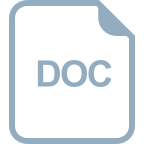
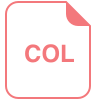
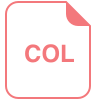
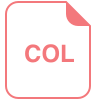
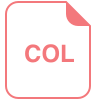
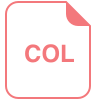






