C语言实现一个可增加长度的链表
时间: 2024-09-28 22:07:31 浏览: 28
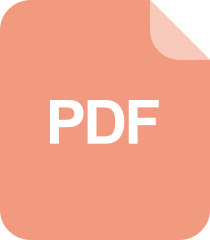
C语言实现输出链表中倒数第k个节点

在C语言中,我们可以使用结构体和指针来实现一个动态增长的链表。这里是一个简单的例子,定义了一个链表节点(`struct Node`),并提供了一个`LinkedList`类型的结构体来维护链表的头结点和一些基本操作。
```c
#include <stdio.h>
#include <stdlib.h>
// 链表节点结构
typedef struct Node {
int data; // 节点的数据
struct Node* next; // 指向下一个节点的指针
} Node;
// 动态链表结构
typedef struct LinkedList {
Node* head; // 链表头节点
int size; // 当前链表元素个数
} LinkedList;
// 初始化链表
LinkedList* createList() {
LinkedList* list = (LinkedList*)malloc(sizeof(LinkedList));
list->head = NULL;
list->size = 0;
return list;
}
// 在链表末尾添加元素
void append(LinkedList* list, int value) {
if (list->size == 0) {
list->head = (Node*)malloc(sizeof(Node));
list->head->data = value;
list->head->next = NULL;
} else {
Node* newNode = (Node*)malloc(sizeof(Node));
newNode->data = value;
newNode->next = NULL;
Node* temp = list->head;
while (temp->next != NULL) {
temp = temp->next;
}
temp->next = newNode;
}
list->size++;
}
// 打印链表
void printList(LinkedList* list) {
Node* temp = list->head;
while (temp != NULL) {
printf("%d -> ", temp->data);
temp = temp->next;
}
printf("NULL\n");
}
int main() {
LinkedList* list = createList();
append(list, 1);
append(list, 2);
append(list, 3);
printList(list); // 输出: 1 -> 2 -> 3 -> NULL
return 0;
}
```
在这个例子中,`createList()` 函数用于初始化一个新的链表,`append()`函数用于在链表末尾添加新元素,`printList()`则用于遍历并打印链表的内容。链表的长度会随着`append()`的调用来动态增长。
阅读全文
相关推荐
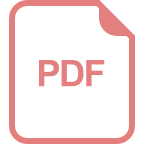
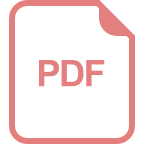
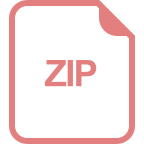
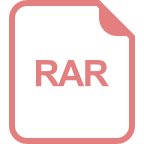
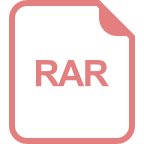
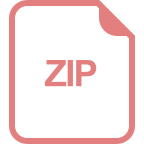
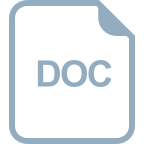
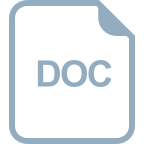
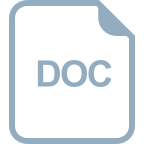
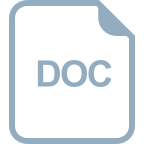
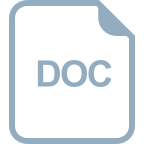
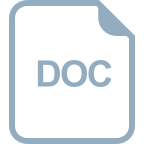
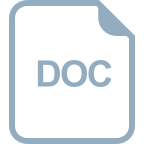
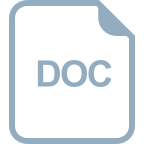
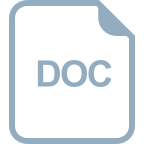
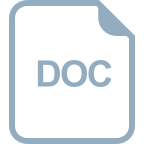

