python实现DH算法
时间: 2024-10-25 10:02:13 浏览: 47
Diffie-Hellman (DH) 算法是一种用于密钥交换的非对称加密协议,它允许两个通信方在没有预先共享秘密的情况下安全地创建一个共同的秘密密钥。Python中可以使用内置的`cryptography`库来实现DH算法。以下是基本步骤:
1. 导入必要的模块:
```python
from cryptography.hazmat.primitives.asymmetric import dh
from cryptography.hazmat.primitives.kdf.pbkdf2 import PBKDF2HMAC
from cryptography.hazmat.primitives import hashes, serialization
```
2. 生成 Diffie-Hellman 公钥对:
```python
generator = dh.generate(2048) # 生成一个2048位素数群的生成元
public_numbers = generator.public_key()
private_key = generator.private_key()
```
3. 分别将公钥发送给对方:
```python
public_key_bytes = public_numbers.public_bytes(
encoding=serialization.Encoding.Raw,
format=serialization.PublicFormat.Raw
)
```
4. 接收方接收并计算共享密钥:
```python
received_public_key = ... # 接收到对方的公钥
shared_key = private_key.exchange(received_public_key)
```
5. 使用 KDF(如PBKDF2)对共享密钥进行进一步处理,增加安全性:
```python
salt = b'some_salt' # 加盐值
kdf = PBKDF2HMAC(
algorithm=hashes.SHA256(),
length=32, # 选择合适的密钥长度
salt=salt,
iterations=100000,
)
derived_key = kdf.derive(shared_key)
```
阅读全文
相关推荐














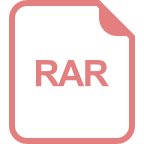



