(三)Linux的文件I/O操作
时间: 2024-02-07 09:02:13 浏览: 44
Linux的文件I/O操作可以使用系统调用来实现。其中,常用的系统调用有open、read、write、close等。
open是打开文件的系统调用,可以指定文件的路径、打开模式等参数。例如,打开一个文件并返回一个文件描述符的代码如下:
```c
#include <stdio.h>
#include <fcntl.h>
#include <unistd.h>
int main() {
int fd;
fd = open("test.txt", O_RDONLY);
if(fd == -1) {
perror("open");
return 1;
}
printf("File descriptor: %d\n", fd);
return 0;
}
```
read是读取文件的系统调用,可以指定读取的文件描述符、缓冲区地址、读取的字节数等参数。例如,读取一个文件并输出其内容的代码如下:
```c
#include <stdio.h>
#include <fcntl.h>
#include <unistd.h>
#define BUF_SIZE 1024
int main() {
int fd, n;
char buf[BUF_SIZE];
fd = open("test.txt", O_RDONLY);
if(fd == -1) {
perror("open");
return 1;
}
while((n = read(fd, buf, BUF_SIZE)) > 0) {
write(STDOUT_FILENO, buf, n);
}
close(fd);
return 0;
}
```
write是写入文件的系统调用,可以指定写入的文件描述符、缓冲区地址、写入的字节数等参数。例如,将用户输入的内容写入一个文件的代码如下:
```c
#include <stdio.h>
#include <fcntl.h>
#include <unistd.h>
#define BUF_SIZE 1024
int main() {
int fd, n;
char buf[BUF_SIZE];
fd = open("test.txt", O_WRONLY | O_CREAT | O_TRUNC, 0644);
if(fd == -1) {
perror("open");
return 1;
}
while((n = read(STDIN_FILENO, buf, BUF_SIZE)) > 0) {
write(fd, buf, n);
}
close(fd);
return 0;
}
```
close是关闭文件的系统调用,可以指定要关闭的文件描述符。例如,关闭一个文件的代码如下:
```c
#include <stdio.h>
#include <fcntl.h>
#include <unistd.h>
int main() {
int fd;
fd = open("test.txt", O_RDONLY);
if(fd == -1) {
perror("open");
return 1;
}
// do something
close(fd);
return 0;
}
```
相关推荐
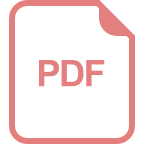
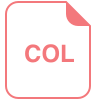
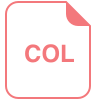
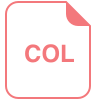
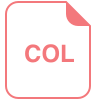
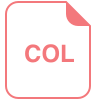









