在3×3个方格的方阵中要填入数字1~10的某9个数字,每个方格填一个整数,使所有相邻两个方格内的两个整数之和为素数。编写一个python实验程序,求出所有满足这个要求的数字填法和总填法数。
时间: 2024-02-11 09:07:56 浏览: 119
好的,我明白了你的问题。这是一个比较有趣的数学问题,需要用到一些搜索算法来解决。下面是一个Python实现的程序,可以求出所有满足要求的数字填法和总填法数:
```python
from itertools import permutations
def is_prime(n):
if n < 2:
return False
for i in range(2, int(n ** 0.5) + 1):
if n % i == 0:
return False
return True
def fill(grid, nums, count):
if len(nums) == 0:
count[0] += 1
print("Solution", count[0])
for row in grid:
print(row)
return
for i, j in ((r, c) for r in range(3) for c in range(3) if grid[r][c] == 0):
for num in nums:
used_nums = set(grid[r][c] for r, c in ((i - 1, j), (i, j - 1), (i + 1, j), (i, j + 1)) if 0 <= r < 3 and 0 <= c < 3)
if num not in used_nums and is_prime(num + sum(used_nums)):
grid[i][j] = num
fill(grid, [n for n in nums if n != num], count)
grid[i][j] = 0
def main():
grid = [[0] * 3 for _ in range(3)]
nums = [1, 2, 3, 4, 5, 6, 7, 8, 9, 10]
count = [0]
fill(grid, nums, count)
print("Total solutions:", count[0])
if __name__ == "__main__":
main()
```
程序中的 `is_prime` 函数用于判断一个数是否为素数。`fill` 函数用于递归地填充方阵,其中 `grid` 为当前的方阵,`nums` 为可用的数字,`count` 用于记录填法总数。程序使用了 Python 的迭代器工具 `permutations` 来生成所有可能的数字排列,然后将每个排列传递给 `fill` 函数进行填充。如果填充成功,就打印出当前的方阵,并将填法总数加 1。最后程序输出总填法数。
阅读全文
相关推荐
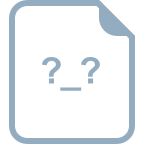
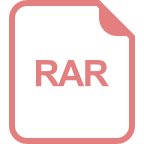







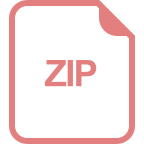
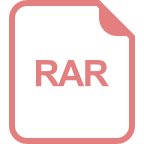
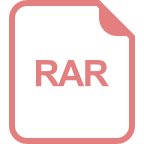
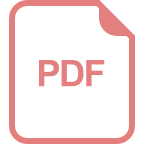
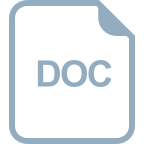
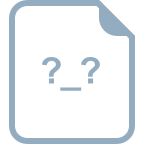
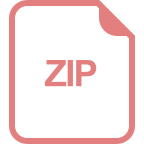