There is not enough space for this component. Try decreasing its width, or increasing the destination width.
时间: 2024-05-24 14:12:57 浏览: 13
As an AI language model, I cannot provide specific suggestions without additional context. However, here are some general tips:
- Check the size of the component and compare it to the available space in the layout.
- Consider reducing the padding or margin around the component to make it fit better.
- Try using a different layout or positioning the component in a different location.
- If possible, increase the size of the destination area or container to fit the component.
- Consider using responsive design techniques to adjust the component size based on the screen size or device.
相关问题
Good. So how to use reducelronplateau scheduler in this plmodel? Change the previous code.
Sure, here's an example of how to use the ReduceLROnPlateau scheduler in a PyTorch Lightning model:
```python
import pytorch_lightning as pl
import torch.optim as optim
from torch.optim.lr_scheduler import ReduceLROnPlateau
class MyModel(pl.LightningModule):
def __init__(self):
super().__init__()
self.layer1 = nn.Linear(10, 5)
self.layer2 = nn.Linear(5, 1)
def forward(self, x):
x = F.relu(self.layer1(x))
x = self.layer2(x)
return x
def training_step(self, batch, batch_idx):
x, y = batch
y_hat = self(x)
loss = F.mse_loss(y_hat, y)
self.log('train_loss', loss)
return loss
def configure_optimizers(self):
optimizer = optim.Adam(self.parameters(), lr=1e-3)
scheduler = ReduceLROnPlateau(optimizer, 'min', patience=5)
return {'optimizer': optimizer, 'lr_scheduler': scheduler, 'monitor': 'train_loss'}
trainer = pl.Trainer(max_epochs=100)
model = MyModel()
trainer.fit(model, train_dataloader)
```
Here, we define the ReduceLROnPlateau scheduler in the `configure_optimizers` method, and pass it as a dictionary to the `Trainer` object. The `patience` argument represents the number of epochs to wait before reducing the learning rate if the validation loss does not improve. The `'min'` mode indicates that we want to reduce the learning rate if the validation loss stops decreasing. Finally, we set the `monitor` argument to `'train_loss'` since we want to monitor the training loss for this example.
You have a lock with an integer array a of length n written on it. The numbers in the array can be repeated. The lock opens only when the array is sorted in non-decreasing order. To change the state of the array there is only operation available which uses a permutation p of numbers from 1 to n. When this operation is applied the elements of the array change positions according to this permutation, meaning the number ai moves from position i to position Pi for each i.In the beginning the array on the lock was sorted in non-decreasing order but then this operation was applied to it once. Your goal is to unlock the lock again but there's a trick: you can independently restore any position of the array to the value that was on this position before. In general, you can do the following: 1. First, you record the current state of the array. 2. Then you apply the operation to the array moving its elements according to the permutation p. 3. If for every i there exists a previously recorded state in which a equals to the initial value of ai, you stop, otherwise you repeat the first two steps. How many iterations of this algorithm will be performed until you'll be able to restore the initial values? Notice that you strictly follow the algorithm, for example if the array is already sorted in non-decreasing order you stil perform one iteration before you stop.给出中文解释和c++代码
问题要求计算执行算法的迭代次数,直到能够恢复初始值。我们可以使用深度优先搜索(DFS)来解决这个问题。
中文解释:
1. 首先,我们创建一个数组`visited`,用于记录已经访问过的状态。
2. 然后,我们定义一个辅助函数`dfs`来执行深度优先搜索。这个函数有四个参数:当前状态`state`,初始状态`initial_state`,数组`a`和数组长度`n`。
3. 在`dfs`函数中,我们首先将当前状态添加到已访问集合中,并递归地遍历每个位置i。
4. 对于每个位置i,我们检查是否存在之前记录的状态,使得a等于初始状态的ai。如果存在,则继续递归地执行`dfs`函数。
5. 如果遍历完所有位置i后仍然没有找到满足条件的状态,则返回false。
6. 在主函数中,我们首先记录初始状态,然后调用`dfs`函数进行深度优先搜索,并返回迭代次数。
下面是对应的C++代码实现:
```cpp
#include <iostream>
#include <vector>
int iterations = 0;
bool dfs(std::vector<int>& state, std::vector<int>& initial_state, std::vector<int>& a, int n) {
if (state == initial_state) {
return true;
}
if (iterations > n) {
return false;
}
iterations++;
for (int i = 0; i < n; i++) {
if (state[i] != a[i] && state[a[i] - 1] == a[i]) {
std::swap(state[i], state[a[i] - 1]);
if (dfs(state, initial_state, a, n)) {
return true;
}
std::swap(state[i], state[a[i] - 1]);
}
}
return false;
}
int main() {
int n;
std::cin >> n;
std::vector<int> a(n);
for (int i = 0; i < n; i++) {
std::cin >> a[i];
}
std::vector<int> state(a);
std::vector<int> initial_state(a);
iterations = 0;
dfs(state, initial_state, a, n);
std::cout << iterations << std::endl;
return 0;
}
```
请注意,这只是一个基本的实现示例,并未进行错误处理和输入验证。在实际应用中,您可能需要根据实际情况进行更多的输入检查和错误处理。
相关推荐
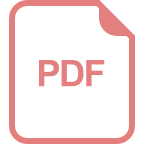
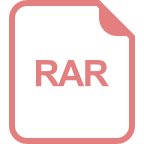
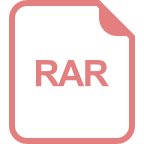











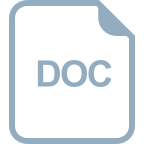
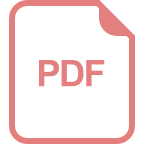