用python根据cv2.canny边缘检测编写一个函数
时间: 2023-05-27 09:07:55 浏览: 143
以下是使用Python编写的基本的cv2.canny边缘检测函数:
```python
import cv2
def cannyEdgeDetection(image, minVal, maxVal):
# 转换为灰度图像
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# 使用高斯滤波器平滑图像
blurred = cv2.GaussianBlur(gray, (3, 3), 0)
# 使用Canny算法检测边缘
edges = cv2.Canny(blurred, minVal, maxVal)
return edges
```
其中,函数的参数包括:
- `image`:需要进行边缘检测的原始图像;
- `minVal`:Canny算法中的低阈值,用于控制边缘的检测灵敏度;
- `maxVal`:Canny算法中的高阈值,用于控制边缘的检测精度。
函数中的操作包括:
1. 将图像转换为灰度图像;
2. 使用高斯滤波器平滑图像,以消除噪声;
3. 使用Canny算法检测边缘。
最终返回的是边缘检测结果。
相关问题
python手动实现cv2.canny算子
好的,下面是Python手动实现cv2.canny算子的代码:
```python
import cv2
import numpy as np
def canny(image, low_threshold, high_threshold):
# Convert the image to grayscale
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# Apply Gaussian blur to the grayscale image
blur = cv2.GaussianBlur(gray, (5, 5), 0)
# Apply the Sobel operator to the blurred image
sobelx = cv2.Sobel(blur, cv2.CV_64F, 1, 0, ksize=3)
sobely = cv2.Sobel(blur, cv2.CV_64F, 0, 1, ksize=3)
# Compute the gradient magnitude and direction
mag = np.sqrt(sobelx**2 + sobely**2)
theta = np.arctan2(sobely, sobelx)
# Convert the gradient direction to degrees
angle = np.rad2deg(theta)
# Apply non-maximum suppression
nms = np.zeros_like(mag)
for i in range(1, nms.shape[0]-1):
for j in range(1, nms.shape[1]-1):
if (angle[i,j] <= 22.5 and angle[i,j] > -22.5) or (angle[i,j] <= -157.5 and angle[i,j] > 157.5):
if mag[i,j] > mag[i,j-1] and mag[i,j] > mag[i,j+1]:
nms[i,j] = mag[i,j]
elif (angle[i,j] <= 67.5 and angle[i,j] > 22.5) or (angle[i,j] <= -112.5 and angle[i,j] > -157.5):
if mag[i,j] > mag[i-1,j-1] and mag[i,j] > mag[i+1,j+1]:
nms[i,j] = mag[i,j]
elif (angle[i,j] <= 112.5 and angle[i,j] > 67.5) or (angle[i,j] <= -67.5 and angle[i,j] > -112.5):
if mag[i,j] > mag[i-1,j] and mag[i,j] > mag[i+1,j]:
nms[i,j] = mag[i,j]
elif (angle[i,j] <= 157.5 and angle[i,j] > 112.5) or (angle[i,j] <= -22.5 and angle[i,j] > -67.5):
if mag[i,j] > mag[i-1,j+1] and mag[i,j] > mag[i+1,j-1]:
nms[i,j] = mag[i,j]
# Apply hysteresis thresholding
edges = np.zeros_like(nms)
edges[nms > high_threshold] = 255
edges[nms < low_threshold] = 0
for i in range(1, edges.shape[0]-1):
for j in range(1, edges.shape[1]-1):
if edges[i,j] == 255:
if edges[i-1,j-1] == 0:
edges[i-1,j-1] = 128
if edges[i-1,j] == 0:
edges[i-1,j] = 128
if edges[i-1,j+1] == 0:
edges[i-1,j+1] = 128
if edges[i,j-1] == 0:
edges[i,j-1] = 128
if edges[i,j+1] == 0:
edges[i,j+1] = 128
if edges[i+1,j-1] == 0:
edges[i+1,j-1] = 128
if edges[i+1,j] == 0:
edges[i+1,j] = 128
if edges[i+1,j+1] == 0:
edges[i+1,j+1] = 128
edges[edges == 128] = 255
edges[edges != 255] = 0
return edges.astype(np.uint8)
```
其中,参数`image`为待处理的图像,`low_threshold`和`high_threshold`分别为低阈值和高阈值。返回值为处理后的二值图像。
该函数的实现过程如下:
1. 将图像转换为灰度图像;
2. 对灰度图像进行高斯模糊;
3. 对模糊后的图像进行Sobel算子计算,得到水平方向和垂直方向的梯度;
4. 计算每个像素点的梯度幅值和梯度方向;
5. 对梯度方向进行非极大值抑制,保留每个方向上梯度幅值最大的像素点;
6. 对抑制后的图像进行双阈值边缘连接,将高于高阈值的像素点视为强边缘,低于低阈值的像素点视为背景,介于两者之间的像素点视为弱边缘;
7. 对弱边缘像素点进行连接,将其与其相邻的强边缘像素点连接起来,最终得到二值图像。
需要注意的是,该函数的实现并不能完全等同于cv2.canny函数的实现,因为在非极大值抑制和边缘连接的过程中可能存在一些差异。
cv2.Canny():边缘检测的用法
cv2.Canny() 是 OpenCV 库中用于边缘检测的函数。它可以帮助我们找到图像中的边缘,并对其进行高亮显示。
函数的语法如下:
```
edges = cv2.Canny(image, threshold1, threshold2, apertureSize, L2gradient)
```
参数说明:
- `image`:输入图像,通常为灰度图像。
- `threshold1`:第一个阈值,用于边缘强度的梯度值低于此阈值时将被认为不是边缘。
- `threshold2`:第二个阈值,用于边缘强度的梯度值高于此阈值时将被认为是边缘。
- `apertureSize`:Sobel 算子的孔径大小,默认为 3。
- `L2gradient`:一个布尔值,指定计算梯度幅值的方法。如果为 True,则使用 L2 范数;如果为 False,则使用 L1 范数。默认为 False。
函数返回一个包含边缘信息的二进制图像,其中边缘点被设置为白色,非边缘点被设置为黑色。
示例代码:
```python
import cv2
# 读取图像
image = cv2.imread('image.jpg', 0)
# 边缘检测
edges = cv2.Canny(image, 100, 200)
# 显示结果
cv2.imshow('Edges', edges)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
这是一个简单的示例,展示了如何使用 cv2.Canny() 函数进行边缘检测。你可以根据实际需求调整阈值和其他参数来获取更好的结果。
阅读全文
相关推荐
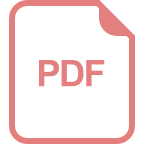
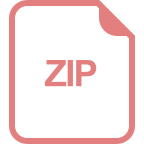
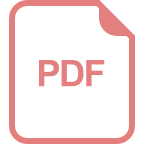
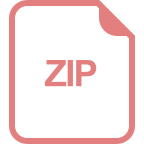





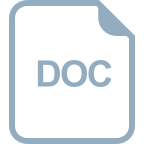
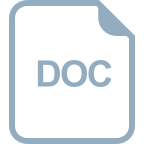




