安卓 java实现扫雷app 代码 包括xml
时间: 2024-03-26 09:35:16 浏览: 26
好的,以下是一个简单的扫雷游戏的Java代码和XML布局示例,供您参考:
Java代码:
```java
public class MainActivity extends AppCompatActivity {
private int[][] board; // 存储雷区的数据
private boolean[][] revealed; // 存储每个格子是否被翻开
private boolean[][] flagged; // 存储每个格子是否被标记为雷
private int numMines; // 雷的数量
private TextView tvMines; // 显示剩余雷的数量的TextView
private GridLayout grid; // 显示游戏区域的GridLayout
private int numRows = 10; // 行数
private int numCols = 10; // 列数
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_main);
// 初始化雷区数据
board = new int[numRows][numCols];
revealed = new boolean[numRows][numCols];
flagged = new boolean[numRows][numCols];
// 随机生成雷的位置
for (int i = 0; i < numMines; i++) {
int row = (int) (Math.random() * numRows);
int col = (int) (Math.random() * numCols);
if (board[row][col] == -1) {
i--;
} else {
board[row][col] = -1; // -1表示这个格子是雷
}
}
// 计算每个格子周围的雷的数量
for (int row = 0; row < numRows; row++) {
for (int col = 0; col < numCols; col++) {
if (board[row][col] != -1) {
int count = 0;
for (int r = row - 1; r <= row + 1; r++) {
for (int c = col - 1; c <= col + 1; c++) {
if (r >= 0 && r < numRows && c >= 0 && c < numCols && board[r][c] == -1) {
count++;
}
}
}
board[row][col] = count; // 数字表示周围的雷的数量
}
}
}
// 初始化游戏区域
grid = findViewById(R.id.grid);
for (int row = 0; row < numRows; row++) {
for (int col = 0; col < numCols; col++) {
Button button = new Button(this);
button.setTag(R.id.row, row);
button.setTag(R.id.col, col);
button.setOnClickListener(new View.OnClickListener() {
@Override
public void onClick(View v) {
int row = (int) v.getTag(R.id.row);
int col = (int) v.getTag(R.id.col);
reveal(row, col);
}
});
button.setOnLongClickListener(new View.OnLongClickListener() {
@Override
public boolean onLongClick(View v) {
int row = (int) v.getTag(R.id.row);
int col = (int) v.getTag(R.id.col);
flag(row, col);
return true;
}
});
grid.addView(button);
}
}
// 更新雷的数量的TextView
tvMines = findViewById(R.id.tvMines);
tvMines.setText(getString(R.string.mines, numMines));
}
// 翻开一个格子
private void reveal(int row, int col) {
if (revealed[row][col] || flagged[row][col]) {
return;
}
revealed[row][col] = true;
Button button = (Button) grid.getChildAt(row * numCols + col);
button.setEnabled(false);
if (board[row][col] == -1) {
// 触雷,游戏结束
button.setText("*");
Toast.makeText(this, "Game Over", Toast.LENGTH_SHORT).show();
} else if (board[row][col] == 0) {
// 翻开周围的格子
for (int r = row - 1; r <= row + 1; r++) {
for (int c = col - 1; c <= col + 1; c++) {
if (r >= 0 && r < numRows && c >= 0 && c < numCols) {
reveal(r, c);
}
}
}
} else {
// 显示周围的雷的数量
button.setText(String.valueOf(board[row][col]));
}
}
// 标记一个格子为雷
private void flag(int row, int col) {
if (revealed[row][col]) {
return;
}
flagged[row][col] = !flagged[row][col];
Button button = (Button) grid.getChildAt(row * numCols + col);
if (flagged[row][col]) {
button.setText("F");
} else {
button.setText("");
}
tvMines.setText(getString(R.string.mines, numMines - countFlags()));
}
// 计算已标记为雷的数量
private int countFlags() {
int count = 0;
for (int row = 0; row < numRows; row++) {
for (int col = 0; col < numCols; col++) {
if (flagged[row][col]) {
count++;
}
}
}
return count;
}
}
```
XML布局:
```xml
<GridLayout
android:id="@+id/grid"
android:layout_width="match_parent"
android:layout_height="match_parent"
android:columnCount="10"
android:rowCount="10"
android:orientation="horizontal"
android:useDefaultMargins="true">
</GridLayout>
<TextView
android:id="@+id/tvMines"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="@string/mines"
android:textSize="24sp"
android:layout_gravity="center_horizontal"
android:layout_marginTop="16dp"
android:layout_marginBottom="16dp"/>
```
以上代码仅供参考,可能存在漏洞和不足之处,需要您自行完善和调试。
相关推荐
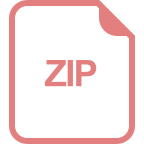
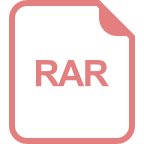
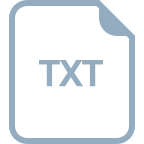
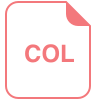
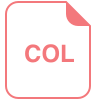
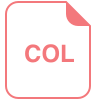
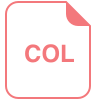
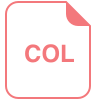









