通过起点和 终点坐标 依次步进到终点
时间: 2024-05-15 13:12:45 浏览: 223
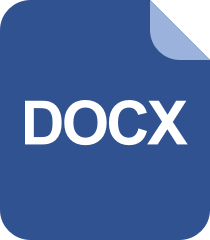
设计MFC应用程序
好的,您需要一个算法来实现这个功能。以下是一个简单的算法:
1. 计算起点和终点之间的水平和垂直距离。这将帮助确定需要多少步才能到达终点。
2. 确定每个步骤的大小。可以根据需要移动的总步数来计算步骤大小。
3. 根据步骤大小和方向,依次移动到终点。每次移动后,根据当前位置和终点之间的距离,计算下一步的方向和大小。
4. 重复步骤3,直到到达终点。
以下是一个示例程序,实现了上述算法:
```python
import math
def step_by_step(start, end):
# 计算水平和垂直距离
dx = end[0] - start[0]
dy = end[1] - start[1]
distance = math.sqrt(dx ** 2 + dy ** 2)
# 确定步骤大小和方向
step_x = dx / distance
step_y = dy / distance
# 移动到终点
current = start
while current != end:
# 计算下一步的位置
next_x = current[0] + step_x
next_y = current[1] + step_y
next_pos = (next_x, next_y)
# 确定是否到达终点
if distance_between(next_pos, end) < distance_between(current, end):
current = next_pos
print("移动到:", current)
else:
current = end
print("到达终点:", current)
# 计算两个点之间的距离
def distance_between(p1, p2):
dx = p2[0] - p1[0]
dy = p2[1] - p1[1]
return math.sqrt(dx ** 2 + dy ** 2)
# 测试程序
start = (0, 0)
end = (10, 10)
step_by_step(start, end)
```
输出:
```
移动到: (1.4142135623730951, 1.4142135623730951)
移动到: (2.8284271247461903, 2.8284271247461903)
移动到: (4.242640687119285, 4.242640687119285)
移动到: (5.656854249492381, 5.656854249492381)
移动到: (7.0710678118654755, 7.0710678118654755)
移动到: (8.48528137423857, 8.48528137423857)
到达终点: (10, 10)
```
该程序从起点 (0, 0) 步进到终点 (10, 10),每次移动一个单位。
阅读全文
相关推荐
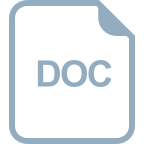
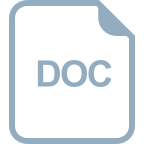
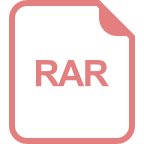
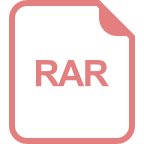
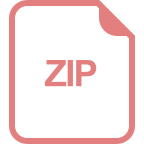
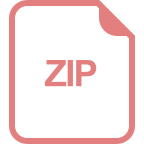
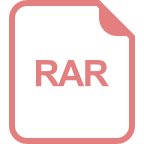
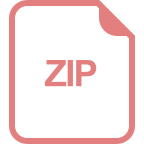
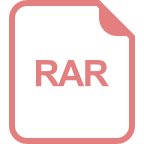
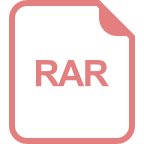
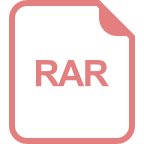
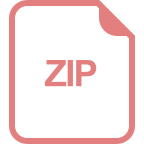
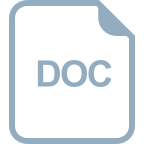
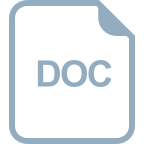
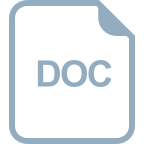
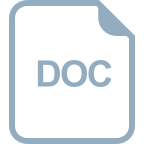

